LZW压缩算法
作者:追风剑情 发布于:2022-1-20 15:21 分类:C#
- using System;
- using System.Text;
- using System.Collections;
- using System.Collections.Generic;
- using System.IO;
- namespace LZWTest
- {
- class Program
- {
- static void Main(string[] args)
- {
- string test = "ABABCDCDABCD";
- Console.WriteLine("测试字符串 {0}", test);
- LZW lzw = new LZW();
- List<ushort> compress = lzw.Encode(test);
- Console.WriteLine();
- Console.WriteLine("压缩列表");
- for (int i = 0; i < compress.Count; i++)
- Console.Write(compress[i] + " ");
- Console.WriteLine();
- string str = lzw.Decode(compress);
- Console.WriteLine();
- Console.WriteLine("方式一 解压 {0}", str);
- byte[] compressBytes = lzw.GetCompressData();
- string str1 = lzw.Decode(compressBytes);
- Console.WriteLine("方式二 解压 {0}", str);
- Console.Read();
- }
- }
- /// <summary>
- /// 串表压缩算法(LZW)
- /// 用12位来表示可能的字符串或字符,前256代表单字符,剩下的3840给可能出现的字符组合
- ///
- /// GIF规范
- /// 1.原始数据用8位表示
- /// 2.标号用9位-12位表示(256-4096)
- /// 3.标号一开始用9位表示(256-512),当达到512时再用10位表示,依次类推
- /// 4.当达到12位能表示的最大数时(4096),在这里插入清除标志,又从9位开始
- /// 5.清除标志(clear flag)的值是原始数据字长表示的最大值加1
- /// 6.结束标志(end flag)的值是原始数据字长表示的最大值加2
- /// </summary>
- public sealed class LZW
- {
- //清除标志
- private ushort clearFlag = 256;
- //结束标志
- private ushort endFlag = 257;
- //字典最大长度
- private int maxDicCount = 4096;
- //存储压缩后的bit流
- private MemoryStream compressStream;
- private BinaryWriter compressWriter;
- private byte[] compressData;
- //初始化标号字典
- private void InitCodeDic(Dictionary<ZString, ushort> codeDic)
- {
- codeDic.Clear();
- //原始数据设置到标号字典中
- for (ushort i = 0; i <= 255; i++)
- {
- char c = (char)i;
- ZString key = new ZString(c);
- codeDic.Add(key, i);
- }
- }
- //编码
- public List<ushort> Encode(string str)
- {
- compressStream = new MemoryStream();
- compressWriter = new BinaryWriter(compressStream);
- //输出列表
- List<ushort> output = new List<ushort>();
- //查询表<前缀字符串, 编码>
- Dictionary<ZString, ushort> codeDic = new Dictionary<ZString, ushort>();
- //前缀
- ZString prefix = null;
- //后缀
- ZString suffix = null;
- //标号
- ushort mark = 258;
- InitCodeDic(codeDic);
- if (prefix == null)
- prefix = new ZString();
- Console.WriteLine("建立字典");
- ushort code;
- for (int i=0; i<str.Length; i++)
- {
- char c = str[i];
- if (prefix.IsNullOrEmpty())
- {
- prefix.Add(c);
- continue;
- }
- suffix = new ZString(c);
- ZString key = prefix + suffix;
- if (codeDic.ContainsKey(key))
- {
- prefix = key;
- }
- else
- {
- codeDic.Add(key, mark);
- mark++;
- code = codeDic[prefix];
- output.Add(code);
- Console.WriteLine("{0}->{1}", prefix, code);
- prefix = suffix;
- WriteCompressStream(code, 12, compressWriter);
- //字典长度已达上限
- if (codeDic.Count >= maxDicCount)
- {
- //插入清除标志
- output.Add(clearFlag);
- InitCodeDic(codeDic);
- prefix.Clear();
- mark = 258;
- WriteCompressStream(clearFlag, 12, compressWriter);
- }
- }
- }
- //考虑最后一个字符
- code = codeDic[prefix];
- output.Add(code);
- //插入结束标志
- output.Add(endFlag);
- Console.WriteLine("{0}->{1}", prefix, code);
- WriteCompressStream(endFlag, 12, compressWriter);
- BinaryReader br = new BinaryReader(compressStream);
- compressData = br.ReadBytes((int)compressStream.Length);
- return output;
- }
- //获取压缩后的数据
- public byte[] GetCompressData()
- {
- return compressData;
- }
- //解码 (按照单字符8bit,组合字符12bit解码)
- public string Decode(byte[] bytes)
- {
- //转成bit数组
- BitArray bitArray = new BitArray(bytes);
- //输出列表
- List<byte> output = new List<byte>();
- //查询表<编码,字符串>
- Dictionary<ushort, ZString> codeDic = new Dictionary<ushort, ZString>();
- ZString prefix = new ZString();
- ushort mark = 258;
- int bitIndex = 0;
- while (bitIndex < bitArray.Length)
- {
- ushort code = bitArray.GetUShort(bitIndex, 12);
- if (code <= 255)
- {
- output.Add((byte)code);
- prefix.Add(code);
- if (prefix.Count == 1)
- continue;
- //前缀为多字符时加入字典 (重建字典)
- if (!codeDic.ContainsKey(mark))
- {
- codeDic.Add(mark, prefix);
- prefix = new ZString(code);
- mark++;
- }
- continue;
- }
- else if (code == clearFlag)
- {
- codeDic.Clear();
- prefix.Clear();
- mark = 258;
- }
- else if (code == endFlag)
- {
- break;
- }
- else if (codeDic.ContainsKey(code))
- {
- prefix = codeDic[code];
- output.AddRange(prefix.ToArray());
- mark++;
- continue;
- }
- else
- {
- Console.WriteLine("重建字典出错,未找到 code={0} 对应的子串", code);
- }
- }
- return Encoding.ASCII.GetString(output.ToArray());
- }
- //解码
- public string Decode(List<ushort> list)
- {
- //输出列表
- List<byte> output = new List<byte>();
- //查询表<编码,字符串>
- Dictionary<ushort, ZString> codeDic = new Dictionary<ushort, ZString>();
- ZString prefix = new ZString();
- ushort mark = 258;
- for (ushort i=0; i<list.Count; i++)
- {
- ushort code = list[i];
- //原始ASCII字符直接输出
- if (code <= 255)
- {
- output.Add((byte)code);
- prefix.Add(code);
- if (prefix.Count == 1)
- continue;
- //前缀为多字符时加入字典 (重建字典)
- if (!codeDic.ContainsKey(mark))
- {
- codeDic.Add(mark, prefix);
- prefix = new ZString(code);
- mark++;
- }
- continue;
- }
- else if (code == clearFlag)
- {
- codeDic.Clear();
- prefix.Clear();
- mark = 258;
- continue;
- }
- else if (code == endFlag)
- {
- break;
- }
- else if (codeDic.ContainsKey(code))
- {
- prefix = codeDic[code];
- output.AddRange(prefix.ToArray());
- mark++;
- continue;
- }
- else
- {
- //如果执行到这里,说明动态重建查询表出了问题
- Console.WriteLine("无法查询到 code={0} 对应的字符串", code);
- }
- }
- return Encoding.ASCII.GetString(output.ToArray());
- }
- //将数据写入压缩流
- private void WriteCompressStream(ushort value, int bitSize, BinaryWriter bw)
- {
- BitArray arr = new BitArray(new int[1] { value });
- for (int i = 0; i < bitSize; i++)
- {
- bw.Write(arr[i]);
- }
- bw.Flush();
- }
- public class ZString
- {
- private List<byte> list = new List<byte>();
- public ZString() { }
- public ZString(char c) { Add(c); }
- public ZString(string s) { Add(s); }
- public ZString(int i) { Add(i); }
- public ZString(byte b) { Add(b); }
- public int Count { get { return list.Count; } }
- public byte[] ToArray()
- {
- return list.ToArray();
- }
- public bool IsNullOrEmpty()
- {
- return list.Count == 0;
- }
- public void Add(byte[] bytes)
- {
- if (bytes == null)
- return;
- list.AddRange(bytes);
- }
- public void Add(int i)
- {
- list.Add((byte)i);
- }
- public void Add(char c)
- {
- byte b = (byte)c;
- list.Add(b);
- }
- public void Add(byte b)
- {
- list.Add(b);
- }
- public void Add(string str)
- {
- if (string.IsNullOrEmpty(str))
- return;
- for (int i = 0; i < str.Length; i++)
- Add(str[i]);
- }
- public void Clear()
- {
- list.Clear();
- }
- public override int GetHashCode()
- {
- //BKDR Hash
- ulong hash = 0;
- //也可以乘以31、131、1313、13131、131313..
- uint p = 1313;
- for (int i=0; i<list.Count; i++)
- {
- //当作p进制计算
- hash = hash * p + list[i];
- }
- return (int)hash;
- }
- public override bool Equals(object obj)
- {
- ZString key = obj as ZString;
- if (key == null)
- return false;
- return GetHashCode() == key.GetHashCode();
- }
- public override string ToString()
- {
- return Encoding.ASCII.GetString(list.ToArray());
- }
- public static ZString operator +(ZString a, ZString b)
- {
- ZString zs = new ZString();
- zs.Add(a.ToArray());
- zs.Add(b.ToArray());
- return zs;
- }
- public static ZString operator +(ZString a, char b)
- {
- ZString zs = new ZString();
- zs.Add(a.ToArray());
- zs.Add(b);
- return zs;
- }
- }
- }
- }
- public static class BitArrayExtension
- {
- public static int GetInt(this BitArray array, int startIndex, int bitLength)
- {
- var newArray = new BitArray(bitLength);
- for (int i = 0; i < bitLength; i++)
- {
- if (array.Length <= startIndex + i)
- {
- newArray[i] = false;
- }
- else
- {
- bool bit = array.Get(startIndex + i);
- newArray[i] = bit;
- }
- }
- return newArray.ToInt();
- }
- public static int ToInt(this BitArray array)
- {
- if (array == null)
- {
- Console.WriteLine("array is nothing.");
- return 0;
- }
- if (array.Length > 32)
- {
- Console.WriteLine("must be at most 32 bits long.");
- return 0;
- }
- var result = new int[1];
- array.CopyTo(result, 0);
- return result[0];
- }
- public static ushort GetUShort(this BitArray array, int startIndex, int bitLength)
- {
- var newArray = new BitArray(bitLength);
- for (int i = 0; i < bitLength; i++)
- {
- if (array.Length <= startIndex + i)
- {
- newArray[i] = false;
- }
- else
- {
- bool bit = array.Get(startIndex + i);
- newArray[i] = bit;
- }
- }
- return newArray.ToUShort();
- }
- public static ushort ToUShort(this BitArray array)
- {
- if (array == null)
- {
- Console.WriteLine("array is nothing.");
- return 0;
- }
- if (array.Length > 32)
- {
- Console.WriteLine("must be at most 32 bits long.");
- return 0;
- }
- var result = new int[1];
- array.CopyTo(result, 0);
- return (ushort)result[0];
- }
- }
运行效果
标签: C#
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年3月(4)
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
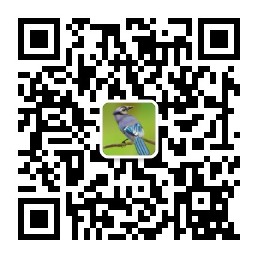