NSFileManager类
作者:追风剑情 发布于:2019-3-4 16:32 分类:Objective-C
Foundation框架允许你利用文件系统对文件或目录执行基本操作。这些基本操作是由NSFileManager类提供的,这个类的方法具有如下功能:
- 创建一个新文件
- 从现有文件中读取数据
- 将数据写入文件
- 重命名文件
- 删除文件
- 测试文件是否存在
- 确定文件的大小和其他属性
- 复制文件
- 测试两个文件的内容是否相同
上面的多数方法也可以对目录进行操作。例如,创建目录、读取目录的内容,或者删除目录。另一个重要特性是链接文件,即同一个文件存在两个不同的名字,有时甚至位于不同的目录中。
使用NSFileHandle类提供的方法,可以打开文件并对文件执行多次读/写操作。NSFileHandle类的方法可以实现如下功能:
- 打开一个文件,执行读、写或更新(读取和写入)操作。
- 在文件中查找指定位置。
- 从文件中读取特定数目的字节,或将指定数目的字节写入文件中。
NSFileHandle类提供的方法也可用于各种设备或套接字。
NSURL类允许在应用中使用URL方法。
NSBundle类提供了允许在应用中使用包(bundle)的方法,包括搜索包中的特定资源(如JPEG图片)。
常用的NSFileManager方法 | |
方法 | 描述 |
-(NSData *) contentsAtPath: path | 从一个文件中读取数据 |
-(BOOL) createFileAtPath: path contents: (NSData *) data attributes: attr | 向一个文件写入数据 |
-(BOOL) removeItemAtPath: path error: err | 删除一个文件 |
-(BOOL) moveItemAtPath: from toPath: to error: err | 重命名或者移动一个文件(to不能是已存在的) |
-(BOOL) copyItemAtPath: from toPath: to error: err | 复制文件(to不能是已存在的) |
-(BOOL) contentsEqualAtPath: path1 andPath: path2 | 比较两个文件的内容 |
-(BOOL) fileExistsAtPath: path | 测试文件是否存在 |
-(BOOL) isReadableFileAtPath: path | 测试文件是否存在,并且是否能执行读操作 |
-(BOOL) isWritableFileAtPath: path | 测试文件是否存在,并且是否能执行写操作 |
-(NSDictionary *) attributesOfItemAtPath: path error: err | 获取文件的属性,所有属性对应的词典中的键都定义在<Foundation/NSFileManager.h>中,例如,表示文件大小的键为NSFileSize |
-(BOOL) setAttributesOfItemAtPath: attr error: err | 更改文件的属性 |
-(NSString *) currentDirectoryPath | 获取当前目录 |
-(BOOL) changeCurrentDirectoryPath: path | 更改当前目录 |
-(BOOL) copyItemAtPath: from toPath: to error: err |
复制目录结构(to不能是已存在的) |
-(BOOL) createDirectoryAtPath: path withIntermediate Directories: (BOOL) flag attributes: attr |
创建一个新目录 |
-(BOOL) fileExistsAtPath: path is Directory: (BOOL *) flag |
测试文件是不是目录(flag中存储结果YES/NO) |
-(NSArray *) contentsOfDirectoryAtPath: path error: err |
列出目录内容 |
-(NSDirectoryEnumerator *) enumeratorAtPath: path |
枚举目录内容 |
-(BOOL) removeItemAtPath: path error: err |
删除空目录 |
-(BOOL) moveItemAtPath: from toPath: to error: err | 重命名或移动一个目录(to不能是已存在的) |
说明:
path、path1、path2、from和to都是NSString对象,attr是一个NSDictionary对象;err是一个指向NSError对象的指针,能提供更多的错误信息。如果err指定为NULL,就会采取默认的行为,具有BOOL返回值的方法,即如果操作成功,就会返回YES;否则就会返回NO。
主目录的完整路径为/users/用户名
特殊的代字符(~)作为用户主目录的缩写。~xxx等价于/users/xxx
Foundation的文件处理不支持其他特殊的UNIX风格,如表示当前目录的“.”和表示父目录的“..”
小知识:
示例一
#import <Foundation/Foundation.h> int main(int argc, const char * argv[]) { @autoreleasepool { NSString *fName = @"testfile"; NSFileManager *fm; NSDictionary *attr; // 需要创建文件管理器的实例 fm = [NSFileManager defaultManager]; NSLog(@"\n currentDirectoryPath: %@", [fm currentDirectoryPath]); // 首先确认测试文件存在 if ([fm fileExistsAtPath: fName] == NO) { NSLog(@"File does't exist"); return 1; } // 创建一个副本 if ([fm copyItemAtPath: fName toPath: @"newfile" error: NULL] == NO) { NSLog(@"File Copy failed!"); return 2; } // 测试两个文件是否一致 if ([fm contentsEqualAtPath: fName andPath: @"newfile"] == NO) { NSLog(@"Files are Not Equal!"); return 3; } // 重命名副本 if ([fm moveItemAtPath: @"newfile" toPath: @"newfile2" error: NULL] == NO) { NSLog(@"File rename Failed"); return 4; } // 获取newfile2的大小 if ((attr = [fm attributesOfItemAtPath: @"newfile2" error: NULL]) == nil) { NSLog(@"Couldn't get file attributes!"); return 5; } NSLog(@"File size is %llu bytes", [[attr objectForKey: NSFileSize] unsignedLongLongValue]); // 最后删除原始文件 if ([fm removeItemAtPath: fName error: NULL] == NO) { NSLog(@"file remove failed"); return 6; } NSLog(@"All operations were successful"); // 显示新创建的文件内容 NSLog(@"%@", [NSString stringWithContentsOfFile: @"newfile2" encoding: NSUTF8StringEncoding error: NULL]); } return 0; }
示例二:目录操作
#import <Foundation/Foundation.h> int main(int argc, const char * argv[]) { @autoreleasepool { NSString *dirName = @"testdir"; NSString *path; NSFileManager *fm; // 需要创建文件管理器的实例 fm = [NSFileManager defaultManager]; // 获取当前目录 path = [fm currentDirectoryPath]; NSLog(@"Current directory path is %@", path); // 创建一个新目录 if ([fm createDirectoryAtPath: dirName withIntermediateDirectories: YES attributes:nil error: NULL] == NO) { NSLog(@"Coundn't create directory!"); return 1; } // 重命名新的目录 if ([fm moveItemAtPath: dirName toPath: @"newdir" error: NULL] == NO) { NSLog(@"Directory rename failed!"); return 2; } // 更改目录到新的目录 if ([fm changeCurrentDirectoryPath: @"newdir"] == NO) { NSLog(@"Change directory failed!"); return 3; } // 获取并显示当前的工作目录 path = [fm currentDirectoryPath]; NSLog(@"Current directory path is %@", path); NSLog(@"All operations were successful!"); } return 0; }
在iOS设备上,程序是运行在沙盒中,如果在设备中运行这个程序,会看到当前目录是/,这说明应用的根目录是在运行它的沙盒中,并不是整个iOS设备文件目录的根。
示例三:枚举目录
#import <Foundation/Foundation.h> int main(int argc, const char * argv[]) { @autoreleasepool { NSString *path; NSFileManager *fm; NSDirectoryEnumerator *dirEnum; NSArray *dirArray; fm = [NSFileManager defaultManager]; // 获取当前工作目录 path = [fm currentDirectoryPath]; // 枚举目录 // enumeratorAtPath 这个方法会递归遍历目录 dirEnum = [fm enumeratorAtPath: path]; NSLog(@"Contents of %@", path); BOOL flag; while ((path = [dirEnum nextObject]) != nil) { NSLog(@"%@", path); // 如果是子目录,则跳过遍历 [fm fileExistsAtPath: path isDirectory: &flag]; if (flag == YES) [dirEnum skipDescendants]; } // 另一种枚举目录的方法,非递归遍历 dirArray = [fm contentsOfDirectoryAtPath: [fm currentDirectoryPath] error: NULL]; NSLog(@"Contents using contentsOfDirectoryAtPath:error:"); //for (path in dirArray) //NSLog(@"%@", path); // 打印数组 NSLog(@"%@", dirArray); } return 0; }
标签: Objective-C
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年11月(3)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
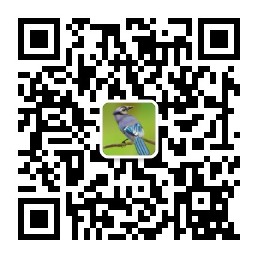