GPU实例化技术(GPU Instancing)
作者:追风剑情 发布于:2018-9-22 16:52 分类:Shader
参考文章 http://www.manew.com/thread-50914-1-1.html
[官方文档] GPU实例化
当场景中有大量使用相同材质和网格的物体时,通过GPU Instancing可以大幅降低Draw Call数量。
示例:创建200个小球,看看开启和不开启GPU Instancing时的draw call数量
下面是一个支持GPU Instancing的简单Shader
// Upgrade NOTE: upgraded instancing buffer 'MyProperties' to new syntax. Shader "Unlit/Sphere" { Properties { _Color("Color", Color) = (1,1,1,1) } SubShader { Tags { "RenderType"="Opaque" "LightMode"="ForwardBase" } LOD 100 Pass { CGPROGRAM #pragma target 3.0 #pragma vertex vert #pragma fragment frag #pragma fragmentoption ARB_precision_hint_fastest #pragma multi_compile_fwdbase //加上这句后,材质球会多出一个Enable GPU Instancing勾选项 #pragma multi_compile_instancing #include "UnityCG.cginc" struct appdata { float4 vertex : POSITION; UNITY_VERTEX_INPUT_INSTANCE_ID }; struct v2f { float4 vertex : SV_POSITION; //只有当你需要在Fragment Shader中访问每个Instance独有的属性时才需要写这个宏。 UNITY_VERTEX_INPUT_INSTANCE_ID }; //float4 _Color; //传统的声明方式不再需要了 //新的声明方式,支持GPU Instancing UNITY_INSTANCING_BUFFER_START(MyProperties) UNITY_DEFINE_INSTANCED_PROP(float4, _Color) #define _Color_arr MyProperties //这句代码是Unity自动插入的 UNITY_INSTANCING_BUFFER_END(MyProperties) v2f vert (appdata v) { v2f o; //这个宏让Instance ID在Shader函数里能够被访问到 UNITY_SETUP_INSTANCE_ID(v); //把Instance ID从输入结构拷贝至输出结构中。 //只有当你需要在Fragment Shader中访问每个Instance独有的属性时才需要写这个宏。 UNITY_TRANSFER_INSTANCE_ID(v,o); o.vertex = UnityObjectToClipPos(v.vertex); return o; } fixed4 frag (v2f i) : SV_Target { //这个宏让Instance ID在Shader函数里能够被访问到 //要想访问独有属性必须调用这个宏 UNITY_SETUP_INSTANCE_ID(i); //访问每个Instance独有的属性 fixed4 col = UNITY_ACCESS_INSTANCED_PROP(_Color_arr, _Color); return col; } ENDCG } } }
下面是一个用来生成小球的脚本
using System.Collections; using System.Collections.Generic; using UnityEngine; public class SphereTest : MonoBehaviour { public int count = 200; public int radius = 10; public GameObject sphere; public List<MeshRenderer> renders = new List<MeshRenderer>(); void Start () { CloneSphere(); SetSphereColor(); } private void CloneSphere() { for (int i=0; i<count; i++) { GameObject go = Instantiate(sphere); MeshRenderer mr = go.GetComponent<MeshRenderer>(); renders.Add(mr); go.transform.localPosition = Random.insideUnitSphere * radius; } } private void SetSphereColor() { for (int i = 0; i < renders.Count; i++) { //SetColor(renders[i]); SetColorPropertyBlock(renders[i]); } } private void SetColor(MeshRenderer mr) { //这种方式会导致创建新的Material mr.material.color = new Color(Random.value, Random.value, Random.value); } private void SetColorPropertyBlock(MeshRenderer mr) { //这种方式不会创建新的Material MaterialPropertyBlock properties = new MaterialPropertyBlock(); properties.SetColor( "_Color", new Color(Random.value, Random.value, Random.value) ); mr.SetPropertyBlock(properties); } }
启用GPU Instancing的运行截图
关闭GPU Instancing的运行截图
使用Instancing的限制
下列情况不能使用Instancing:
(1) 使用Lightmap的物体
(2) 受不同Light Probe / Reflection Probe影响的物体
(3) 使用包含多个Pass的Shader的物体,只有第一个Pass可以Instancing
(4) 前向渲染时,受多个光源影响的物体只有Base Pass可以instancing,Add Passes不行
(5) 另外,由于Constant Buffer的尺寸限制,一个Instanced Draw Call能画的物体数量是有上限的(参见UnityInstancing.cginc中的UNITY_MAX_INSTANCE_COUNT)
最后需要再次强调的是,Instancing在Shader上有额外的开销,并不是总能提高帧率。永远要以实际Profiling的结果为准!
在UnityInstancing.cginc文件中可找到UNITY_MAX_INSTANCE_COUNT的定义
// The maximum number of instances a single instanced draw call can draw. // You can define your custom value before including this file. #ifndef UNITY_MAX_INSTANCE_COUNT #define UNITY_MAX_INSTANCE_COUNT 500 #endif #if (defined(SHADER_API_GLES3) || defined(SHADER_API_GLCORE) || defined(SHADER_API_METAL)) && !defined(UNITY_MAX_INSTANCE_COUNT_GL_SAME) // Many devices have max UBO size of 16kb #define UNITY_INSTANCED_ARRAY_SIZE (UNITY_MAX_INSTANCE_COUNT / 4) #else // On desktop, this assumes max UBO size of 64kb #define UNITY_INSTANCED_ARRAY_SIZE UNITY_MAX_INSTANCE_COUNT #endif
在UnityInstancing.cginc文件中可找到UNITY_VERTEX_INPUT_INSTANCE_ID的定义
// Used in vertex shader input / output struct. #define UNITY_VERTEX_INPUT_INSTANCE_ID uint instanceID : SV_InstanceID;
默认情况下,如果您的场景中没有启用GPU实例化的游戏对象,则Unity会删除实例化着色器变体。要覆盖剥离行为,请设置:
Edit->Project Settings->Graphics->Shader Stripping->Instancing Variants->Keep All。
标签: Shader
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
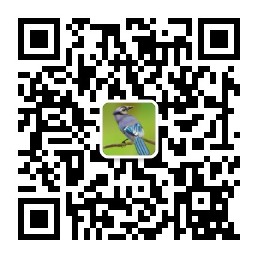