C#将Excel表导出为json文件
作者:追风剑情 发布于:2018-5-26 12:07 分类:C#
示例:Excel表导出为json文件
需要引用两个文件
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Linq;
- using System.Text;
- using System.Threading.Tasks;
- using System.Windows.Forms;
- using System.Diagnostics;
- using System.IO;
- //文档 https://docs.microsoft.com/zh-cn/previous-versions/7fzyhc74%28v%3dvs.110%29
- //引用 C:\Windows\assembly\Microsoft.Office.Tools.Excel.v9.0.dll
- //引用COM Interop.Microsoft.Office.Interop.Excel.dll
- using Excel = Microsoft.Office.Interop.Excel;
- namespace ExcelTool
- {
- public partial class MainForm : Form
- {
- private string configPath;
- public MainForm()
- {
- InitializeComponent();
- Init();
- }
- private void Init()
- {
- this.AllowDrop = true; //允许文件拖入窗口
- this.Load += MainForm_Load;
- this.DragEnter += MainForm_DragEnter;
- this.btnBrowser.MouseUp += btnBrowser_MouseUp;
- this.btnExportJson.MouseUp += btnExportJson_MouseUp;
- }
- private void MainForm_Load(object sender, EventArgs e)
- {
- Console.WriteLine("MainForm_Load");
- }
- private void MainForm_DragEnter(object sender, DragEventArgs e)
- {
- //获取拖进来的文件路径
- configPath = ((System.Array)e.Data.GetData(DataFormats.FileDrop)).GetValue(0).ToString();
- this.textBoxPath.Text = configPath;
- }
- private void btnBrowser_MouseUp(object sender, MouseEventArgs e)
- {
- ShowOpenFileDialog();
- this.textBoxPath.Text = configPath;
- }
- private void btnExportJson_MouseUp(object sender, MouseEventArgs e)
- {
- if (string.IsNullOrWhiteSpace(configPath))
- {
- MessageBox.Show("请先选择要导出的excel文件", "提示");
- return;
- }
- ExportJson();
- }
- private void ShowOpenFileDialog()
- {
- //打开选择文件对话框
- OpenFileDialog file = new OpenFileDialog();
- file.Filter = "Excel(*.xlsx)|*.xlsx|Excel(*.xls)|*.xls";
- file.InitialDirectory = Environment.GetFolderPath(Environment.SpecialFolder.Desktop);
- file.Multiselect = false;
- if (file.ShowDialog() == DialogResult.Cancel)
- return;
- //判断文件后缀
- var path = file.FileName;
- string fileSuffix = System.IO.Path.GetExtension(path);
- if (string.IsNullOrEmpty(fileSuffix))
- return;
- switch (fileSuffix)
- {
- case ".xls": //Excel 97-2003
- break;
- case ".xlsx": //Excel 2007-更高
- break;
- }
- configPath = path;
- }
- private void ExportJson()
- {
- //创建应用
- Excel.Application xlApp = (Excel.Application)(new Excel.ApplicationClass());
- if (xlApp == null)
- {
- MessageBox.Show("不能访问Excel");
- return;
- }
- //判断使用的Excel版本
- if (Convert.ToDouble(xlApp.Version) < 12)
- {
- //Excel 97-2003
- }
- else
- {
- //Excel 2007-更高
- }
- xlApp.Visible = false;
- xlApp.UserControl = true;
- object missing = System.Reflection.Missing.Value;
- //以只读方式打开文件
- Excel.Workbook wb = xlApp.Application.Workbooks.Open(configPath, missing, true, missing, missing, missing, missing, missing, missing, true, missing, missing, missing, missing, missing);
- //获取所有工作表
- Excel.Sheets sheets = wb.Worksheets;
- Excel.Worksheet worksheet;
- for (int i = 1; i <= sheets.Count; i++)
- {
- worksheet = (Excel.Worksheet)sheets[i];
- //构造json
- string json = BuildJson(worksheet);
- //保存文件
- SaveJson(worksheet.Name, json);
- }
- xlApp.Quit();
- xlApp = null;
- //关掉excel进程
- Process[] procs = Process.GetProcessesByName("excel");
- foreach (Process pro in procs)
- {
- pro.Kill();
- }
- GC.Collect();
- MessageBox.Show("导出成功");
- }
- private void SaveJson(string fileName, string jsonStr)
- {
- string path = string.Format("{0}/{1}.txt", Path.GetDirectoryName(configPath), fileName);
- File.WriteAllText(path, jsonStr);
- }
- private string BuildJson(Excel.Worksheet sheet)
- {
- if (null == sheet)
- return "";
- //获取行数、列数
- int usedRowCount = sheet.UsedRange.Cells.Rows.Count;
- int usedColumnCount = sheet.UsedRange.Cells.Columns.Count;
- string[] COLUMN_LETTER = new string[]{
- "A", "B", "C", "D", "E", "F", "G", "H", "I", "J", "K", "L", "M", "N",
- "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z"};
- string C = COLUMN_LETTER[usedColumnCount - 1];
- //获取数据类型定义区(即,第二行)
- Excel.Range typeRange = sheet.Cells.get_Range("A2", C + "2");
- object[,] typeValue2 = (object[,])typeRange.Value2;
- //获取key定义区(即,第三行)
- Excel.Range keyRange = sheet.Cells.get_Range("A3", C + "3");
- object[,] keyValue2 = (object[,])keyRange.Value2;
- //获取数据区(即,排除标题行)
- Excel.Range dataRange = sheet.Cells.get_Range("A4", C + usedRowCount);
- object[,] value2 = (object[,])dataRange.Value2;
- StringBuilder sb = new StringBuilder();
- sb.AppendLine("[");
- string type, k, v;
- for (int i = 1; i <= dataRange.Rows.Count; i++)
- {
- //遍历时跳过ID为空的行
- if (null == value2[i, 1])
- continue;
- v = value2[i, 1].ToString();
- if (string.IsNullOrWhiteSpace(v))
- continue;
- //构造json串
- sb.Append("{");
- for (int j = 1; j <= dataRange.Columns.Count; j++)
- {
- k = keyValue2[1, j].ToString();
- if (value2[i, j] == null)
- v = "";
- else
- v = value2[i, j].ToString();
- type = typeValue2[1, j].ToString();
- switch(type)
- {
- case "int":
- sb.AppendFormat("\"{0}\":{1}", k, v);
- break;
- case "int[]":
- sb.Append(ParseIntArray(k, v));
- break;
- case "json":
- sb.Append(ParseJson(k, v));
- break;
- case "string":
- sb.AppendFormat("\"{0}\":\"{1}\"", k, v);
- break;
- case "string[]":
- sb.Append(ParseStringArray(k, v));
- break;
- }
- if (j < dataRange.Columns.Count)
- sb.Append(",");
- }
- sb.Append("}");
- if (i < dataRange.Rows.Count)
- sb.AppendLine(",");
- }
- sb.AppendLine();
- sb.Append("]");
- return sb.ToString();
- }
- private string ParseIntArray(string k, string v)
- {
- string format = "\"{0}\":[{1}]";
- if (string.IsNullOrWhiteSpace(v))
- {
- return string.Format(format, k, "");
- }
- return string.Format("\"{0}\":[{1}]", k, v);
- }
- private string ParseStringArray(string k, string v)
- {
- string format = "\"{0}\":[{1}]";
- if (string.IsNullOrWhiteSpace(v)) {
- return string.Format(format, k, "");
- }
- StringBuilder sb = new StringBuilder();
- string[] strArr = v.Split(',');
- int len = strArr.Length;
- for (int i=0; i<len; i++)
- {
- sb.AppendFormat("\"{0}\"", strArr[i]);
- if (i < len - 1)
- sb.Append(",");
- }
- return string.Format(format, k, sb.ToString());
- }
- private string ParseJson(string k, string v)
- {
- if (string.IsNullOrWhiteSpace(v))
- return string.Format("\"{0}\":{{}}", k);
- return string.Format("\"{0}\":{1}", k, v);
- }
- }
- }
运行效果
导出文件截图
解决方法
设置嵌入互操作类型为False
如果在C:\Windows下没找到Microsoft.Office.Interop.Excel.dll还可用用NuGet安装
通过NuGet安装Microsoft.Office.Interop.Excel
注意:如果选择的版本与电脑上安装的Excel版本不匹配,运行时会报错,此时只需重新安装下其他版本方可解决。我电脑上安装的是Excel2010,这里我安装的Microsoft.Office.Interop.Excel版本为12,经亲测完美运行。
标签: C#
« 向量与矩阵的乘法
|
搭建开发环境(TS代码编译器)»
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年3月(4)
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
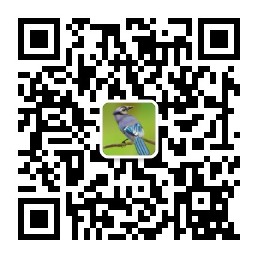