高斯消元法求解方程组(C#实现)
作者:追风剑情 发布于:2017-11-5 12:30 分类:Algorithms
高斯消元法的主要思想是通过对系数矩阵进行行变换,将方程组的系数矩阵由对称矩阵变为三角矩阵,从而达到消元的目的,最后通过回代逐个获得方程组的解。
高斯消元法的实现简单,主要由两个步骤组成:第一个步骤就是通过选择主元,逐行消元,最终形成方程组系数矩阵的三角矩阵形式;第二步骤就是逐步回代的过程,最终矩阵的对角线上的元素就是方程组的解。
- using System;
- using System.Collections.Generic;
- namespace Test4
- {
- class Program
- {
- static void Main(string[] args)
- {
- //测试数据
- /*
- * 2x + 3y + z = 6
- * x - y + 2z = -1
- * x + 2y - z = 5
- */
- float[] matrix = new float[] { 2, 3, 1,
- 1, -1, 2,
- 1, 2, -1};
- float[] value = new float[] { 6, -1, 5 };
- float[] result = GuassEquation.Resolve(matrix, 3, value);
- if (null != result)
- {
- Console.WriteLine("解: x={0}, y={1}, z={2}", result[0], result[1], result[2]);
- }
- Console.Read();
- }
- }
- /// <summary>
- /// 高斯消元法求解方程组
- /// </summary>
- public class GuassEquation
- {
- //矩阵维数
- private static int m_DIM;
- //一维数组形式存放的系数矩阵(必须是方阵)
- private static float[] m_Matrix;
- //方程组的值
- private static float[] m_Value;
- public static float[] Resolve(float[] matrix, int dim, float[] value)
- {
- if (matrix.Length / dim != dim || value.Length != dim)
- {
- throw new ArgumentException("Error, not square matrix.");
- }
- m_Matrix = matrix;
- m_DIM = dim;
- m_Value = value;
- /*消元,得到上三角阵*/
- for(int i = 0; i < m_DIM - 1; i++)
- {
- /*按列选主元*/
- int pivotRow = SelectPivotalElement(i);
- if (pivotRow != i)
- {
- SwapRow(i, pivotRow);
- }
- //主元为0时,不存在唯一解
- if (IsPrecisionZero(i))
- {
- Console.WriteLine("不存在唯一解");
- return null;
- }
- /*对系数归一化处理,使每行的第一个系数是1.0*/
- SimplePivotalRow(i, i);
- /*逐行进行消元*/
- for (int j = i + 1; j < m_DIM; j++)
- {
- RowElimination(i, j);
- }
- }
- /*回代求解*/
- //对最后一个变量求解
- m_Matrix[(m_DIM - 1) * m_DIM + m_DIM - 1] = m_Value[m_DIM - 1] / m_Matrix[(m_DIM - 1) * m_DIM + m_DIM - 1];
- //对剩余变量求解
- for(int i = m_DIM - 2; i >= 0; i--)
- {
- double totalCof = 0.0;
- //将第i行对角线项之后的项相加
- for (int j = i + 1; j < m_DIM; j++)
- {
- totalCof += m_Matrix[i * m_DIM + j] * m_Matrix[j * m_DIM + j];
- }
- //求出对角线上变量的解
- m_Matrix[i * m_DIM + i] = (float)((m_Value[i] - totalCof) / m_Matrix[i * m_DIM + i]);
- }
- /*将对角线元素的解逐个存入解向量*/
- float[] xValue = new float[m_DIM];
- for (int i = 0; i < m_DIM; i++)
- {
- xValue[i] = m_Matrix[i * m_DIM + i];
- }
- return xValue;
- }
- // 判断主元是否为0
- private static bool IsPrecisionZero(int i)
- {
- return m_Matrix[i * m_DIM + i] == 0;
- }
- /// <summary>
- /// 选择主元
- /// 在消元的过程中,如果某一行的对角线元素的值太小,
- /// 在计算过程中就会出现很大的数除以很小的数的情况,
- /// 有除法溢出的可能,因此在消元的过程中,通常都会增加
- /// 一个主元选择的步骤。
- /// 再通过行交换操作,将当前列绝对值最大的行交换到当前位置,
- /// 避免了除法溢出的问题,增加了算法的稳定性。
- /// </summary>
- /// <param name="i">第i行</param>
- /// <returns></returns>
- private static int SelectPivotalElement(int i)
- {
- int col = i;
- int pivotalRow = i;
- float max_val = 0;
- for (; i < m_DIM; i++)
- {
- float val = Math.Abs(m_Matrix[i * m_DIM + col]);
- if (val > max_val)
- {
- max_val = val;
- pivotalRow = i;
- }
- }
- return pivotalRow;
- }
- // 交换行
- private static void SwapRow(int i, int pivotRow)
- {
- for (int j = 0; j < m_DIM; j++)
- {
- float tmp = m_Matrix[i * m_DIM + j];
- m_Matrix[i * m_DIM + j] = m_Matrix[pivotRow * m_DIM + j];
- m_Matrix[pivotRow * m_DIM + j] = tmp;
- }
- float tmp_val = m_Value[i];
- m_Value[i] = m_Value[pivotRow];
- m_Value[pivotRow] = tmp_val;
- }
- // 对系数归一化处理
- private static void SimplePivotalRow(int row, int col)
- {
- for (; row < m_DIM; row++)
- {
- float a = m_Matrix[row * m_DIM + col];
- for (int j = col; j < m_DIM; j++)
- {
- m_Matrix[row * m_DIM + j] /= a;
- }
- m_Value[row] /= a;
- }
- }
- // 消元
- private static void RowElimination(int i, int j)
- {
- int i_start = i * m_DIM;
- int j_start = j * m_DIM;
- //第j行与第i行各项相减
- for (int m = 0; m < m_DIM; m++)
- {
- m_Matrix[j_start + m] -= m_Matrix[i_start + m];
- }
- m_Value[j] -= m_Value[i];
- }
- public static void Print()
- {
- for (int i = 0; i < m_DIM; i++)
- {
- for (int j = 0; j < m_DIM; j++)
- {
- Console.Write(m_Matrix[i * m_DIM + j] + ",");
- }
- Console.WriteLine();
- }
- }
- }
- }
测试
标签: Algorithms
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年3月(4)
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
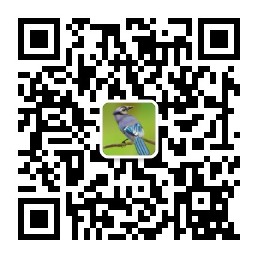