红黑树的旋转操作
作者:追风剑情 发布于:2017-8-19 23:03 分类:Algorithms
参考 http://blog.csdn.net/xudong_98/article/details/51471688
上一篇: 构建一颗红黑树
左旋转与右旋转互为逆操作
示例:C#
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace RedBlackTree1 { class Program { static void Main(string[] args) { int[] keys = new int[] { 13, 8, 1, 11, 6, 17, 15, 25, 22, 27}; Console.Write("测试数据为: "); for (int i = 0; i < keys.Length; i++) { Console.Write(keys[i] + " "); } Console.WriteLine(); //根节点 Node root = null; //录入10个节点 for (int i = 0; i < keys.Length; i++) { Node node = new Node(); node.key = keys[i]; if (null == root) root = node; else Insert(root, node); } Console.Write("构建的二叉搜索树为: "); Print(root); Console.WriteLine(); Console.WriteLine("给二叉搜索树染色: "); Coloring(root, Color.Black);//根节点为黑色 Print(root, true); Console.WriteLine(); Console.WriteLine("对key等于8的结点进行右旋转"); Node node8 = GetNodeByKey(root, 8); RotationRight(node8); Print(root, true); Console.WriteLine(); Console.WriteLine("对key等于1的结点进行左旋转"); Node node1 = GetNodeByKey(root, 1); RotationLeft(node1); Print(root, true); Console.ReadKey(); } static Node GetNodeByKey(Node node, int key) { if (node == null) return null; if (node.key == key) return node; Node left = GetNodeByKey(node.left, key); if (left != null && left.key == key) return left; Node right = GetNodeByKey(node.right, key); if (right != null && right.key == key) return right; return null; } static void Insert(Node root, Node child) { if(root.key >= child.key) { if (null == root.left) { root.left = child; child.parent = root; } else { Insert(root.left, child); } } else { if (null == root.right) { root.right = child; child.parent = root; } else { Insert(root.right, child); } } } // 向左旋转 static void RotationLeft(Node node) { //必须要有右节点 if (node.right == null) return; //轴节点 Node pivot = node; //轴的左节点 Node left = node.left; //轴的右节点 Node right = node.right; //轴的右节点的左节点 Node right_left = right.left; //改变轴的父节点相应指针的指向 Node pivot_parent = pivot.parent; if (null != pivot_parent) { if (pivot_parent.left == pivot) { pivot_parent.left = right; } else if (pivot_parent.right == pivot) { pivot_parent.right = right; } } //右节点的父指针指向轴节点的父 right.parent = pivot_parent; //右节点的左指针指向轴节点 right.left = pivot; //轴节点的父指针指向右节点 pivot.parent = right; //轴节点的右指针指向右节点的左节点 pivot.right = right_left; if (null != right_left) right_left.parent = pivot; } // 向右旋转 static void RotationRight(Node node) { //必须要有左节点 if (node.left == null) return; //轴节点 Node pivot = node; //轴的左节点 Node left = node.left; //轴的右节点 Node right = node.right; //轴的左节点的右节点 Node left_right = left.right; //改变轴的父节点相应指针的指向 Node pivot_parent = pivot.parent; if (null != pivot_parent) { if (pivot_parent.left == pivot) { pivot_parent.left = left; } else if (pivot_parent.right == pivot) { pivot_parent.right = left; } } //左节点的父指针指向轴节点的父 left.parent = pivot_parent; //左节点的右指针指向轴节点 left.right = pivot; //轴节点的父指针指向左节点 pivot.parent = left; //轴节点的左指针指向左节点的右节点 pivot.left = left_right; if (null != left_right) left_right.parent = pivot; } static void Coloring(Node root, Color color) { if (null == root) return; root.color = color; color = (color == Color.Black) ? Color.Red : Color.Black; Coloring(root.left, color); Coloring(root.right, color); } static void Print(Node root, bool outputColor = false) { if (null == root) return; if (root != null) { if (outputColor) Console.Write("{0}({1}) ", root.key, root.color); else Console.Write("{0} ", root.key); } Print(root.left, outputColor); Print(root.right, outputColor); } } public enum Color { Black, Red } public class Node { public Color color; public int key; public Node parent; public Node left; public Node right; } }
绕8号节点右旋后的图像为:
标签: Algorithms
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年11月(3)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
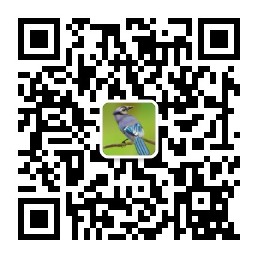