妖怪与和尚过河问题
作者:追风剑情 发布于:2015-11-5 21:20 分类:Algorithms
有三个妖怪和三个和尚要利用唯一一条小船过河,这条小船每次至少载一个人最多载两个人,无论是在河的两岸还是在船上,只要妖怪的数量大于和尚的数量,妖怪们就会将和尚吃掉。现在需要选择一种过河的安排,保证和尚和妖怪都能过河且和尚不能被妖怪吃掉。
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace MonkTest { class Program { static void Main(string[] args) { new AcrossRiver().SearchState(); Console.Read(); } } } /// <summary> /// 过河问题求解类 /// 思路: 把抽象问题转化成计算能处理的数学模型 /// 算法原理:利用状态树求解 (采用深度优先搜索) /// 效率优化: 采用剪枝、去重 /// </summary> public class AcrossRiver { //过河动作枚举 private enum ActionName { //一个妖怪过河 ONE_MONSTER_GO, //两个妖怪过河 TWO_MONSTER_GO, //一个和尚过河 ONE_MONK_GO, //两个和尚过河 TWO_MONK_GO, //一个妖怪和一个和尚过河 ONE_MONSTER_ONE_MONK_GO, //一个妖怪返回 ONE_MONSTER_BACK, //两个妖怪返回 TWO_MONSTER_BACK, //一个和尚返回 ONE_MONK_BACK, //两个和尚返回 TWO_MONK_BACK, //一个妖怪和一个和尚返回 ONE_MONSTER_ONE_MONK_BACK }; //船的行使方向枚举 private enum BoatLocation { REMOTE, //对岸 LOCAL //本岸 } //过河动作数学模型 private class ActionEffection { public ActionName act; public BoatLocation boat_to;//船移动的方向 public int move_monster;//此次移动的妖怪数量 public int move_monk;//此次移动的和尚数量 public ActionEffection(ActionName act, BoatLocation boat_to, int move_monster, int move_monk) { this.act = act; this.boat_to = boat_to; this.move_monster = move_monster; this.move_monk = move_monk; } } private class ItemState { public int local_monster = 3; public int local_monk = 3; public int remote_monster = 0; public int remote_monk = 0; public BoatLocation boat = BoatLocation.LOCAL; public ActionName curAct; public const int MAX_MONSTER_COUNT = 3; public const int MAX_MONK_COUNT = 3; //此方法用于剪枝 public bool CanTakeAction(ActionEffection ae) { if (boat == ae.boat_to) return false; int local_move_monster = local_monster + ae.move_monster; int local_move_monk = local_monk + ae.move_monk; int remote_move_monster = remote_monster - ae.move_monster; int remote_move_monk = remote_monk - ae.move_monk; //数量判断 if (local_move_monster < 0 || local_move_monster > MAX_MONSTER_COUNT) return false; if (local_move_monk < 0 || local_move_monk > MAX_MONK_COUNT) return false; return true; } //判断当前状态是否为最终状态 public bool IsFinalState() { if (remote_monster == MAX_MONSTER_COUNT && remote_monk == MAX_MONK_COUNT) return true; return false; } //判断是否为有效状态 public bool IsValidState() { //如果妖怪数量>=和尚数量,则和尚会被吃掉。 if ((local_monk > 0 && local_monster > local_monk) || (remote_monk > 0 && remote_monster > remote_monk)) return false; return true; } public override string ToString() { string s = string.Format("{0}_{1}_{2}_{3}_{4}", boat, local_monster, local_monk, remote_monster, remote_monk); return s; } public ItemState Clone() { ItemState state = new ItemState(); state.local_monster = local_monster; state.local_monk = local_monk; state.remote_monster = remote_monster; state.remote_monk = remote_monk; state.boat = boat; state.curAct = curAct; return state; } } //过河动作列表 private ActionEffection[] actEffect = new ActionEffection[10]; private StateStack states = new StateStack(); private ItemState last_local_state = null; public AcrossRiver() { //初始化10种过河动作 actEffect[0] = new ActionEffection(ActionName.ONE_MONSTER_GO, BoatLocation.REMOTE, -1, 0); actEffect[1] = new ActionEffection(ActionName.TWO_MONSTER_GO, BoatLocation.REMOTE, -2, 0); actEffect[2] = new ActionEffection(ActionName.ONE_MONK_GO, BoatLocation.REMOTE, 0, -1); actEffect[3] = new ActionEffection(ActionName.TWO_MONK_GO, BoatLocation.REMOTE, 0, -2); actEffect[4] = new ActionEffection(ActionName.ONE_MONSTER_ONE_MONK_GO, BoatLocation.REMOTE, -1, -1); actEffect[5] = new ActionEffection(ActionName.ONE_MONSTER_BACK, BoatLocation.LOCAL, 1, 0); actEffect[6] = new ActionEffection(ActionName.TWO_MONSTER_BACK, BoatLocation.LOCAL, 2, 0); actEffect[7] = new ActionEffection(ActionName.ONE_MONK_BACK, BoatLocation.LOCAL, 0, 1); actEffect[8] = new ActionEffection(ActionName.TWO_MONK_BACK, BoatLocation.LOCAL, 0, 2); actEffect[9] = new ActionEffection(ActionName.ONE_MONSTER_ONE_MONK_BACK, BoatLocation.LOCAL, 1, 1); //初始化栈 states.Push(new ItemState()); } public void SearchState() { ItemState current = states.Back(); if (null == current) return; if (current.IsFinalState()) { states.PrintStack(); return; } //尝试用10种动作分别与当前状态组合 for (int i = 0; i < actEffect.Length; i++) { SearchStateOnNewAction(current, actEffect[i]); } } private void SearchStateOnNewAction(ItemState current, ActionEffection ae) { ItemState nextState = MakeActionNewState(current, ae); if (null != nextState) { if (nextState.IsValidState() && !states.IsProcessedState(nextState)) { states.Push(nextState); SearchState(); states.Pop(); } } } private ItemState MakeActionNewState(ItemState curState, ActionEffection ae) { ItemState newState = null; if (null == last_local_state) { last_local_state = curState; } if (curState.CanTakeAction(ae)) { newState = curState.Clone(); newState.local_monster += ae.move_monster; newState.local_monk += ae.move_monk; newState.remote_monster -= ae.move_monster; newState.remote_monk -= ae.move_monk; newState.boat = ae.boat_to; newState.curAct = ae.act; } return newState; } /// <summary> /// 状态栈 /// </summary> private class StateStack { private List<ItemState> list = new List<ItemState>(); private Dictionary<string, bool> dic = new Dictionary<string, bool>(); private int way = 1; public ItemState Back() { ItemState state = null; if (list.Count > 0) { state = list[list.Count - 1]; } return state; } public void Push(ItemState state) { string key = state.ToString(); if (dic.ContainsKey(key)) return; dic.Add(key, true); list.Add(state); } public void Pop() { if (list.Count > 0) { ItemState state = list[list.Count - 1]; list.RemoveAt(list.Count - 1); dic.Remove(state.ToString()); } } public void Clear() { list.Clear(); dic.Clear(); way = 1; } //判断是否为已搜索过的状态(即,去重) public bool IsProcessedState(ItemState state) { return dic.ContainsKey(state.ToString()); } public void PrintStack() { Console.WriteLine("方案: {0} ({1}步)", way++, list.Count); ItemState state = null; for (int i = 0; i < list.Count; i++) { state = list[i]; Console.WriteLine("{0} ({1}, {2}, {3}, {4})", state.curAct, state.local_monster, state.local_monk, state.remote_monster, state.remote_monk); } Console.WriteLine("end"); } } }
运行效果:
标签: Algorithms
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年11月(3)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
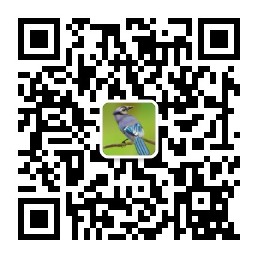