创建和使用数据库
作者:追风剑情 发布于:2015-8-22 18:52 分类:Android
Android使用的是SQLite数据库系统。为一人应用程序所创建的数据库只能被此应用程序访问,其他应用程序将不能访问它。以编程方式创建的SQLite数据库总是存储在/data/data/<package_name>/databases文件夹下。
一、创建数据库辅助类
package com.example.androidtest; import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.SQLException; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import android.util.Log; /** * 访问数据库的辅助类 * 封装了访问数据的所有复杂性。 * 为一个应用程序所创建的数据库只能被此应用程序访问,其他应用程序将不能访问它。 * 数据库文件存储在/data/data/<package_name>/databases文件夹下。 * @author Administrator * */ public class DBAdapter { static final String KEY_ROWID = "_id"; static final String KEY_NAME = "name"; static final String KEY_EMAIL = "email"; static final String TAG = "DBAdapter"; static final String DATABASE_NAME = "MyDB"; static final String DATABASE_TABLE = "contacts"; static final int DATABASE_VERSION = 1; //定义创建表的sql语句常量 static final String DATABASE_CREATE = "create table contacts (_id integer primary key autoincrement, " + "name text not null, email text not null);"; final Context context; DatabaseHelper DBHelper; SQLiteDatabase db; public DBAdapter(Context ctx) { this.context = ctx; DBHelper = new DatabaseHelper(context); } private static class DatabaseHelper extends SQLiteOpenHelper { DatabaseHelper (Context context) { super(context, DATABASE_NAME, null, DATABASE_VERSION); } @Override public void onCreate(SQLiteDatabase db) { try { db.execSQL(DATABASE_CREATE); } catch (SQLException e){ e.printStackTrace(); } } @Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { Log.w(TAG, "Upgrading database from version "+oldVersion + "to " +newVersion+", which will destroy all old data"); //为了简单起见,直接删除现有的表并创建一个新表,在实际中,通常需要备份现在的表, //然后将其内容复制到新表中。 db.execSQL("DROP TABLE IF EXISTS contacts"); onCreate(db); } } /** * 创建or打开数据库 * @return * @throws SQLException */ public DBAdapter open() throws SQLException { db = DBHelper.getWritableDatabase(); return this; } /** * 关闭数据库 */ public void close() { DBHelper.close(); } /** * 插入数据 * @param name * @param email * @return ID */ public long insertContact(String name, String email) { ContentValues initialValues = new ContentValues(); initialValues.put(KEY_NAME, name); initialValues.put(KEY_EMAIL, email); return db.insert(DATABASE_TABLE, null, initialValues); } /** * 删除数据 * @param rowId * @return true:删除成功 */ public boolean deleteContact(long rowId) { return db.delete(DATABASE_TABLE, KEY_ROWID+"="+rowId, null) > 0; } /** * 查询所有数据 * @return Cursor 游标(可看成指向结果集的指针) */ public Cursor getAllContacts() { String[] columns = new String[] {KEY_ROWID, KEY_NAME, KEY_EMAIL}; return db.query(DATABASE_TABLE, columns, null, null, null, null, null); } /** * 查询指定数据 * @param rowId * @return Cursor 游标(可看成指向结果集的指针) * @throws SQLException */ public Cursor getContact(long rowId) throws SQLException { String[] columns = new String[] {KEY_ROWID, KEY_NAME, KEY_EMAIL}; Cursor mCursor = db.query(true, DATABASE_TABLE, columns, KEY_ROWID+"="+rowId, null, null, null, null, null); if(mCursor != null) { mCursor.moveToFirst(); } return mCursor; } /** * 更新指定数据 * @param rowId * @param name * @param email * @return true:更新成功 */ public boolean updateContact(long rowId, String name, String email) { ContentValues args = new ContentValues(); args.put(KEY_NAME, name); args.put(KEY_EMAIL, email); return db.update(DATABASE_TABLE, args, KEY_ROWID+"="+rowId, null) > 0; } }
二、创建活动
视图
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" tools:context="${relativePackage}.${activityClass}" > <Button android:id="@+id/btn_insert" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Insert" android:onClick="onClickInsert" /> <Button android:id="@+id/btn_query_all" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Query all" android:onClick="onClickQueryAll" /> <Button android:id="@+id/btn_query_id" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Query id" android:onClick="onClickQueryID" /> <Button android:id="@+id/btn_update" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Update" android:onClick="onClickUpdate" /> <Button android:id="@+id/btn_delete" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Delete" android:onClick="onClickDelete" /> </LinearLayout>
代码
package com.example.androidtest; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import android.app.Activity; import android.database.Cursor; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Toast; public class DatabasesActivity extends Activity { DBAdapter db; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_databases); db = new DBAdapter(this); db.open(); } @Override protected void onDestroy() { super.onDestroy(); db.close(); } public void onClickInsert(View view) { Log.d("test", "db="+db); long id = db.insertContact("Wei-MengLee", "weimenglee@qq.com"); id = db.insertContact("Mary Jackson", "mary@qq.com"); Toast.makeText(this, "Insert successful", Toast.LENGTH_SHORT).show(); } public void onClickQueryAll(View view) { Cursor c = db.getAllContacts(); if(c.moveToFirst()){ do { DisplayContact(c); } while (c.moveToNext()); } } public void onClickQueryID(View view) { Cursor c = db.getContact(2); if(c.moveToFirst()){ DisplayContact(c); }else{ Toast.makeText(this, "No contact found", Toast.LENGTH_SHORT).show(); } } public void onClickUpdate(View view) { if(db.updateContact(1, "Wei-Meng Lee", "weimenglee@gmail.com")){ Toast.makeText(this, "Update successful", Toast.LENGTH_SHORT).show(); }else{ Toast.makeText(this, "Update failed", Toast.LENGTH_SHORT).show(); } } public void onClickDelete(View view) { if(db.deleteContact(1)){ Toast.makeText(this, "Delete successful", Toast.LENGTH_SHORT).show(); }else{ Toast.makeText(this, "Delete failed", Toast.LENGTH_SHORT).show(); } } public void DisplayContact(Cursor c) { Toast.makeText(this, "id: "+c.getString(0) + "\n" + "Name: "+c.getString(1) + "\n" + "Email: " + c.getString(2), Toast.LENGTH_SHORT).show(); } /** * 把assets目录下的一个现成sqlite文件复制到目标文件夹。 * 注意: assets目录下的文件必须采用小写字母格式。 */ public void CopyMyDB() { try { String destPath = "/data/data/"+getPackageName()+"/databases"; File f = new File(destPath); Log.d("test", "db path="+f.getAbsolutePath()); if (!f.exists()){ f.mkdir(); f.createNewFile(); CopyDB(getBaseContext().getAssets().open("mydb"), new FileOutputStream(destPath+"/MyDB")); } } catch (FileNotFoundException e){ e.printStackTrace(); } catch (IOException e){ e.printStackTrace(); } } public void CopyDB(InputStream inputStream, OutputStream outputStream) throws IOException { byte[] buffer = new byte[1024]; int length; while ((length = inputStream.read(buffer)) > 0) { outputStream.write(buffer, 0, length); } inputStream.close(); outputStream.close(); } }
运行效果
标签: Android
« 基本视图
|
使用内容提供者——Contacts»
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年11月(3)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
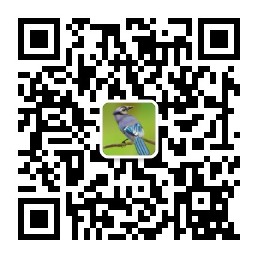