C#哈夫曼编码文件压缩
作者:追风剑情 发布于:2024-10-16 20:43 分类:Algorithms
因为需要将统计表保存到文件中,以便解码时重建哈夫曼树,所以当数据量小时,可能压缩后的文件比原文件还大。哈夫曼编码适合用来压缩数据量大且字符出现频率高的文件。算法原理参见 哈夫曼树(Huffman Tree)
一、哈夫曼算法实现
using System; using System.Collections; using System.Collections.Generic; using System.IO; using System.Text; namespace HuffmanTest { internal class Program { static void Main(string[] args) { //读取测试数据 string s = File.ReadAllText("test.txt"); //压缩 string base64 = HuffmanCoding.Compress(s); File.WriteAllText("encode.txt", base64); //解压 s = HuffmanCoding.Decompress(base64); File.WriteAllText("decode.txt", s); Console.ReadKey(); } }
using System; using System.Collections; using System.Collections.Generic; using System.Text; using System.IO; /// <summary> /// 哈夫曼编码压缩算法 /// </summary> public sealed class HuffmanCoding { //哈夫曼树节点 public sealed class Node : IComparable<Node> { //值 public byte value; //出现频率 public int frequency; public Node left; public Node right; public Node(byte value, int frequency) { this.value = value; this.frequency = frequency; } public int CompareTo(Node other) { return frequency.CompareTo(other.frequency); } } // 生成统计表 private static Dictionary<byte, Node> GenerateStatistics(byte[] data) { //统计不同字节出现的频率 var statistics = new Dictionary<byte, Node>(); foreach (byte b in data) { if (statistics.ContainsKey(b)) statistics[b].frequency++; else statistics.Add(b, new Node(b, 1)); } return statistics; } // 生成哈夫曼编码表 private static Dictionary<byte, string> GenerateCodes(Node node, string code = "", Dictionary<byte, string> codeTable = null) { if (codeTable == null) codeTable = new Dictionary<byte, string>(); //叶子节点 if (node.left == null && node.right == null) { codeTable[node.value] = code; return codeTable; } //树的广度遍历 GenerateCodes(node.left, code + "0", codeTable); GenerateCodes(node.right, code + "1", codeTable); return codeTable; } // 生成啥夫曼树 private static Node GenerateTree(Dictionary<byte, Node> statistics) { List<Node> nodes = new List<Node>(); nodes.AddRange(statistics.Values); nodes.Sort(); //构造哈夫曼树 while (nodes.Count > 1) { //取出频率最小的两个节点 var leftNode = nodes[0]; var rightNode = nodes[1]; nodes.RemoveRange(0, 2); //构造一棵子树 var node = new Node(0, leftNode.frequency + rightNode.frequency); node.left = leftNode; node.right = rightNode; //将子树加入列表并重新排序 nodes.Add(node); nodes.Sort(); } //哈夫曼树的根节点 var rootNode = nodes[0]; return rootNode; } // 压缩数据, 返回 base64 public static string Compress(string s) { byte[] bytes = Encoding.UTF8.GetBytes(s); byte[] encodeData = HuffmanCoding.Compress(bytes); string base64 = Convert.ToBase64String(encodeData); return base64; } // 压缩数据 public static byte[] Compress(byte[] data) { if (data == null) return data; //频率统计表 var statistics = GenerateStatistics(data); //哈夫曼树根节点 var rootNode = GenerateTree(statistics); //哈夫曼编码表 var codeTable = GenerateCodes(rootNode); PrintCodeTable("code_table.txt", codeTable); //生成压缩数据 byte[] encodeData = Encode(statistics, codeTable, data); return encodeData; } // 解压数据 public static string Decompress(string base64) { byte[] bytes = Convert.FromBase64String(base64); byte[] decodeData = HuffmanCoding.Decompress(bytes); return Encoding.UTF8.GetString(decodeData); } // 解压数据 public static byte[] Decompress(byte[] data) { if (data == null) return data; MemoryStream ms = new MemoryStream(data); BinaryReader br = new BinaryReader(ms); //频率统计表 var statistics = ReadStatistics(br); //哈夫曼树根节点 var rootNode = GenerateTree(statistics); //解码数据 var decodeData = Decode(br, rootNode); return decodeData; } // 从内存流读统计表 private static Dictionary<byte, Node> ReadStatistics(BinaryReader br) { var statistics = new Dictionary<byte, Node>(); //读统计表长度 int length = br.ReadInt32(); for (int i = 0; i < length; i++) { byte b = br.ReadByte(); int f = br.ReadInt32(); var node = new Node(b, f); statistics.Add(b, node); } return statistics; } // 解码内容 private static byte[] Decode(BinaryReader br, Node rootNode) { //内容长度 int length = br.ReadInt32(); //压缩数据 var data = br.ReadBytes(length); //数据解码 Node node = rootNode; List<byte> result = new List<byte>(); BitArray bits = new BitArray(data); for (int i = 0; i < bits.Length; i++) { bool b = bits[i]; node = b ? node.right : node.left; //如果是叶子节点 if (node.left == null && node.right == null) { result.Add(node.value); //继续从根节点查找叶子 node = rootNode; //内容已全部解码 if (result.Count >= length) break; } } return result.ToArray(); } // 写统计表到内存流 private static void WriteStatistics(Dictionary<byte, Node> statistics, BinaryWriter bw) { //写入统计表长度 bw.Write(statistics.Count); foreach (byte k in statistics.Keys) { //字节 bw.Write(k); //出现的频率 bw.Write(statistics[k].frequency); } } // 写内容到内存流 private static void WriteData(Dictionary<byte, Node> statistics, Dictionary<byte, string> codeTable, byte[] data, BinaryWriter bw) { //写入内容长度 bw.Write(data.Length); //计算需要用多少bit来存储经哈夫曼编码后的数据 int bitCount = 0; foreach (var b in statistics.Keys) { int frequence = statistics[b].frequency; string code = codeTable[b]; bitCount += code.Length * frequence; } //将数据的哈夫曼编码存储到BitArray中 BitArray bitArray = new BitArray(bitCount); int i = 0; foreach (var b in data) { string code = codeTable[b]; foreach (var c in code) { bitArray.Set(i++, c.Equals('1')); } } //将BitArray中的数据复制到buffer中 int size = bitArray.Count / 8; if (bitArray.Count % 8 > 0) size++; byte[] buffer = new byte[size]; bitArray.CopyTo(buffer, 0); //将编码后的数据写入内存流 bw.Write(buffer); } // 生成编码后的数据 private static byte[] Encode(Dictionary<byte, Node> statistics, Dictionary<byte, string> codeTable, byte[] data) { MemoryStream ms = new MemoryStream(); BinaryWriter bw = new BinaryWriter(ms); WriteStatistics(statistics, bw); WriteData(statistics, codeTable, data, bw); byte[] bytes = ms.ToArray(); return bytes; } // 输出编码表 private static void PrintCodeTable(string filePath, Dictionary<byte, string> codeTable) { StringBuilder sb = new StringBuilder(); foreach (var b in codeTable.Keys) { sb.AppendLine(string.Format("{0}: {1}", (char)b, codeTable[b])); } File.WriteAllText(filePath, sb.ToString()); } }
二、运行测试
标签: Algorithms
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年10月(3)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
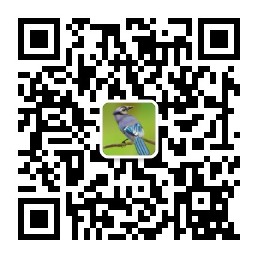