StreamingAssetsLoader
作者:追风剑情 发布于:2024-6-19 15:29 分类:Unity3d
- using System;
- using System.Collections;
- using System.Collections.Generic;
- using System.IO;
- using UnityEngine;
- using UnityEngine.Events;
- using UnityEngine.Serialization;
- /// <summary>
- /// 资源加载器
- /// 从 Application.streamingAssetsPath 下加载文件
- /// </summary>
- public class StreamingAssetsLoader : MonoBehaviour
- {
- //文件url列表,只填写相对路径
- [SerializeField]
- private List<string> m_urls = new List<string>();
- //是否执行Awake方法时自动开始加载
- [SerializeField]
- private bool m_LoadOnAwake = false;
- public string url { get; private set; }
- public string error { get; private set; }
- public float progress { get; private set; }
- public AssetObject asset { get; private set; }
- [Serializable]
- public class FileProgressEvent : UnityEvent<StreamingAssetsLoader> { }
- [Serializable]
- public class FileCompletedEvent : UnityEvent<StreamingAssetsLoader> { }
- [Serializable]
- public class FileErrorEvent : UnityEvent<StreamingAssetsLoader> { }
- [Serializable]
- public class ListCompletedEvent : UnityEvent<StreamingAssetsLoader> { }
- //当前文件加载进度
- [FormerlySerializedAs("onFileProgress")]
- [SerializeField]
- private FileProgressEvent m_OnFileProgress = new FileProgressEvent();
- //当前文件加载完成
- [FormerlySerializedAs("onFileCompleted")]
- [SerializeField]
- private FileCompletedEvent m_OnFileCompleted = new FileCompletedEvent();
- //所有文件加载完成
- [FormerlySerializedAs("onListCompleted")]
- [SerializeField]
- private ListCompletedEvent m_OnListCompleted = new ListCompletedEvent();
- //当前文件加载出错
- [FormerlySerializedAs("onFileError")]
- [SerializeField]
- private FileErrorEvent m_OnFileError = new FileErrorEvent();
- public FileProgressEvent OnFileProgress => m_OnFileProgress;
- public FileCompletedEvent OnFileCompleted => m_OnFileCompleted;
- public ListCompletedEvent OnListCompleted => m_OnListCompleted;
- public FileErrorEvent OnFileError => m_OnFileError;
- //资源类型
- public enum AssetType
- {
- None = 0,//未知
- Text, //文本
- Texture,//图片
- Audio, //音频
- AssetBundle, //资源包
- }
- //资源对象
- public class AssetObject
- {
- public AssetType type;
- public string url;
- public string text;
- public Texture2D texture;
- public AudioClip audioClip;
- public AssetBundle assetBundle;
- public void Dispose()
- {
- texture = null;
- audioClip = null;
- assetBundle = null;
- }
- }
- private void Awake()
- {
- if (m_LoadOnAwake)
- StartLoad();
- }
- // 清空url
- public void Clear()
- {
- m_urls.Clear();
- if (asset != null)
- {
- asset.Dispose();
- asset = null;
- }
- }
- // 设置urls
- public void SetUrls(List<string> urls)
- {
- m_urls = urls;
- }
- // 添加url
- public void AddUrl(string url)
- {
- m_urls.Add(url);
- }
- // 启动加载
- public void StartLoad()
- {
- StartCoroutine(LoadAsync());
- }
- // 停止加载
- public void StopLoad()
- {
- StopCoroutine(LoadAsync());
- }
- private IEnumerator LoadAsync()
- {
- yield return null;
- int index = 0;
- while (index < m_urls.Count)
- {
- url = m_urls[index++];
- url = string.Format("{0}/{1}", Application.streamingAssetsPath, url);
- Debug.LogFormat("Load: {0}", url);
- WWW www = new WWW(url);
- while (!www.isDone)
- {
- progress = www.progress;
- m_OnFileProgress?.Invoke(this);
- yield return www;
- }
- if (!string.IsNullOrEmpty(www.error))
- {
- error = www.error;
- m_OnFileError?.Invoke(this);
- yield break;
- }
- AssetObject obj = new AssetObject();
- obj.url = url;
- obj.type = GetAssetTypeByExtension(url);
- ReadFromWWW(obj, www);
- asset = obj;
- www.Dispose();
- m_OnFileCompleted?.Invoke(this);
- yield return new WaitForEndOfFrame();
- }
- m_OnListCompleted?.Invoke(this);
- }
- private void OnDestroy()
- {
- StopAllCoroutines();
- }
- // 从www中读取资源
- private void ReadFromWWW(AssetObject obj, WWW www)
- {
- switch (obj.type)
- {
- case AssetType.Text:
- obj.text = www.text;
- break;
- case AssetType.Texture:
- obj.texture = www.texture;
- break;
- case AssetType.Audio:
- obj.audioClip = www.GetAudioClip();
- break;
- case AssetType.AssetBundle:
- obj.assetBundle = www.assetBundle;
- break;
- }
- }
- // 通过后缀判断资源类型
- private AssetType GetAssetTypeByExtension(string url)
- {
- string ext = Path.GetExtension(url).ToLower();
- if (ext.Equals(".tif") || ext.Equals(".jpg") || ext.Equals(".png"))
- return AssetType.Texture;
- if (ext.Equals(".txt") || ext.Equals(".json") || ext.Equals(".xml"))
- return AssetType.Text;
- if (ext.Equals(".wav") || ext.Equals(".mp3"))
- return AssetType.Audio;
- //资源包文件后缀,根据需求定义
- if (ext.Equals(".model"))
- return AssetType.AssetBundle;
- return AssetType.None;
- }
- }
标签: Unity3d
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年3月(4)
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
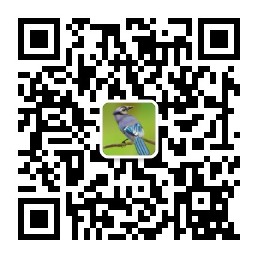