C# WebSocket 服务器实现
作者:追风剑情 发布于:2024-1-15 16:54 分类:C#
Writing WebSocket server
RFC-6455.pdf
通过客户端发送 HTTP GET 请求将连接升级到 WebSocket。
using System; using System.Net.Sockets; using System.Net; using System.Text; using System.Text.RegularExpressions; using System.Security.Cryptography; namespace WebSocketServerTest { /// <summary> /// https://developer.mozilla.org/en-US/docs/Web/API/WebSockets_API/Writing_WebSocket_server /// https://datatracker.ietf.org/doc/html/rfc6455 /// </summary> internal class Program { static void Main(string[] args) { TcpListener server = new TcpListener(IPAddress.Parse("127.0.0.1"), 80); server.Start(); Console.WriteLine("Server has started on 127.0.0.1:80.{0}Waiting for a connection…", Environment.NewLine); TcpClient client = server.AcceptTcpClient(); Console.WriteLine("A client connected."); NetworkStream stream = client.GetStream(); while (true) { while (!stream.DataAvailable); while (client.Available < 3); byte[] bytes = new byte[client.Available]; stream.Read(bytes, 0, bytes.Length); String data = Encoding.UTF8.GetString(bytes); //握手的完整过程参见 RFC 6455 第4.2.2节 //1.获取 "Sec-WebSocket-Key" 请求标头的值,不包含任何前导或尾随空格 //2.将其与 "258EAFA5-E914-47DA-95CA-C5AB0DC85B11" (RFC 6455指定的特殊GUID) 连接 //3.计算新值的 SHA-1 和 Base64 哈希 //4.将散列写回HTTP响应中 "Sec-WebSocket-Accept" 响应标头的值 if (Regex.IsMatch(data, "^GET", RegexOptions.IgnoreCase)) { Console.WriteLine("收到来自客户端的握手请求"); //HTTP/1.1 将序列 CR LF 定义为行尾标记 const string eol = "\r\n"; //从请求头中提取 Sec-WebSocket-Key string swk = new System.Text.RegularExpressions.Regex("Sec-WebSocket-Key: (.*)").Match(data).Groups[1].Value.Trim(); //连接特殊GUID string swka = swk + "258EAFA5-E914-47DA-95CA-C5AB0DC85B11"; //计算新值的 SHA-1 byte[] swkaSha1 = SHA1.Create().ComputeHash(Encoding.UTF8.GetBytes(swka)); //计算新值的 Base64 string swkaSha1Base64 = Convert.ToBase64String(swkaSha1); //返回值 byte[] response = Encoding.UTF8.GetBytes("HTTP/1.1 101 Switching Protocols" + eol + "Connection: Upgrade" + eol + "Upgrade: websocket" + eol + "Sec-WebSocket-Accept: " + swkaSha1Base64 + eol + eol); //返回后代表握手完成! stream.Write(response, 0, response.Length); } else { // 收到来自客户端的WebSocket消息! //fin: 0表示还有后续帧数据;1表示当前帧数据已经是全部数据 bool fin = (bytes[0] & 0b10000000) != 0; bool mask = (bytes[1] & 0b10000000) != 0;//1:表示存在掩蔽密钥 //opcode说明: //0:表示连续帧 //1:表示文本数据 //2:表示二进制数据 //3-7:保留 //8:表示连接关闭 //9:表示ping //0xA:表示pong //oxB-F:保留 int opcode = bytes[0] & 0b00001111; int offset = 2; ulong msglen = bytes[1] & (ulong)0b01111111; //126表示消息长度占2个字节 if (msglen == 126) { //websocket 采用的是大端排列(Big-Endian) //在windows平台上需要转成小端(little-endian) msglen = BitConverter.ToUInt16(new byte[] { bytes[3], bytes[2] }, 0); offset = 4; } //127表示消息长度占8个字节 else if (msglen == 127) { msglen = BitConverter.ToUInt64(new byte[] { bytes[9], bytes[8], bytes[7], bytes[6], bytes[5], bytes[4], bytes[3], bytes[2] }, 0); offset = 10; } if (msglen == 0) { Console.WriteLine("msglen == 0"); } else if (mask) { byte[] decoded = new byte[msglen]; byte[] masks = new byte[4] { bytes[offset], bytes[offset + 1], bytes[offset + 2], bytes[offset + 3] }; offset += 4; for (ulong i = 0; i < msglen; ++i) decoded[i] = (byte)(bytes[(ulong)offset + i] ^ masks[i % 4]); string text = Encoding.UTF8.GetString(decoded); Console.WriteLine("{0}", text); } else { Console.WriteLine("mask bit not set"); Console.WriteLine(); } } } } } }
HTML 测试页面
<!doctype html> <html lang="en"> <style> textarea { vertical-align: bottom; } #output { overflow: auto; } #output > p { overflow-wrap: break-word; } #output span { color: blue; } #output span.error { color: red; } </style> <body> <h2>WebSocket Test</h2> <textarea cols="60" rows="6"></textarea> <button>send</button> <div id="output"></div> </body> <script> // http://www.websocket.org/echo.html const button = document.querySelector("button"); const output = document.querySelector("#output"); const textarea = document.querySelector("textarea"); const wsUri = "ws://127.0.0.1/"; const websocket = new WebSocket(wsUri); button.addEventListener("click", onClickButton); websocket.onopen = (e) => { writeToScreen("CONNECTED"); doSend("WebSocket rocks"); }; websocket.onclose = (e) => { writeToScreen("DISCONNECTED"); }; websocket.onmessage = (e) => { writeToScreen(`<span>RESPONSE: ${e.data}</span>`); }; websocket.onerror = (e) => { writeToScreen(`<span class="error">ERROR:</span> ${e.data}`); }; function doSend(message) { writeToScreen(`SENT: ${message}`); websocket.send(message); } function writeToScreen(message) { output.insertAdjacentHTML("afterbegin", `<p>${message}</p>`); } function onClickButton() { const text = textarea.value; text && doSend(text); textarea.value = ""; textarea.focus(); } </script> </html>
以下为收到的浏览器握手请求数据
GET / HTTP/1.1
Host: 127.0.0.1
Connection: Upgrade
Pragma: no-cache
Cache-Control: no-cache
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/120.0.0.0 Safari/537.36 Edg/120.0.0.0
Upgrade: websocket
Origin: null
Sec-WebSocket-Version: 13
Accept-Encoding: gzip, deflate, br
Accept-Language: zh-CN,zh;q=0.9,en;q=0.8,en-GB;q=0.7,en-US;q=0.6
Sec-WebSocket-Key: f4QQK6qAaBZB44T+xUb54Q==
Sec-WebSocket-Extensions: permessage-deflate; client_max_window_bits
例如 ws://127.0.0.1/test
服务器收到的GET为:
GET /test HTTP/1.1
......
// 从 http get 请求头中解析出url路径
public static string ParseURLPath(string getHeader)
{
Match match = Regex.Match(getHeader, @"GET (.+) HTTP/1.1");
if (!match.Success)
return string.Empty;
string[] arr = match.Value.Split(new char[] { ' ' });
//string path = arr[1].TrimStart('/');
string path = arr[1];
return path;
}
标签: C#
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年10月(4)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
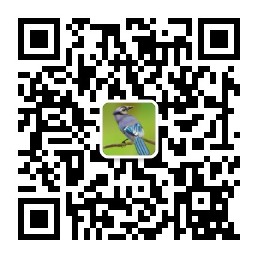