XR Interaction Toolkit
作者:追风剑情 发布于:2022-7-22 17:37 分类:Unity3d
参考文档
XR Interaction Toolkit Manual
XR Interaction Toolkit Examples
Unity - Manual: XR Input
Unity - Packages: Input System
什么是XR?
扩展现实(Extended Reality,简称XR),是AR(增强现实)、VR(虚拟现实)、MR(混合现实)等多种技术的统称。
一个高级的,基于组件的交互系统,用于创建VR和AR体验。它提供了一个框架,以及一个将这两种类型的组件连接在一起的交互管理器。它还包含了您可以用于运动和绘图视觉效果的组件。
XR Origin 组件
Tracking Origin Mode
○ Not Specified:使用输入设备的默认跟踪原点模式。
○ Device: 输入设备将相对于第一个已知位置进行跟踪。
○ Floor:输入设备将相对于地板上的位置进行跟踪。
抓取交互
需要在希望被抓取的对象上挂 XRGrabInteractable 脚本。找到 XR Origin/Camera Offset/LeftHand Controller 对象上的 XRRayInteractor 脚本上的 Force Grab 属性,当此属性为true时,对象将被瞬移到手上。当此属性为false时,对象将被固定在手柄射线的端点上。
相互作用事件(Interactor Events)
XRRayInteractor 脚本上找到 Interactor Events 属性,展开后可以设置相互作用事件回调。手柄进入事件(Hover Entered)、手柄离开事件(Hover Exited)、抓取事件(Select Entered)、释放事件(Select Exited)。
触觉事件(Haptic Events)
XRRayInteractor 脚本上找到 Haptic Events 属性,展开后可以为一些事件设置振动参数。
手柄交互
手柄交互由 XR Origin/Camera Offset/LeftHand Controller 上挂的 ActionBasedController 组件控制。ActionBasedController 负责将从XR输入子系统(XR input subsystem)中获取到的特征值转成XR交互状态(XR interaction state),例如 “选中”,以及控制设备(如,手柄)与控制模型之间的姿态同步。
判断Trigger键是否按下
ActionBasedController rightHandController;
//只会在按下那一刻返回true
if (rightHandController.activateAction.action.triggered)
{
Debug.Log("按下手柄上的Trigger键");
}
判断Trigger键当前动作阶段(Phase)
ActionBasedController rightHandController;
InputActionPhase phase = rightHandController.activateAction.action.phase;
switch(phase)
{
case InputActionPhase.Disabled:
material.SetColor("_Color", Color.red);
break;
case InputActionPhase.Waiting://Trigger键未按下
material.SetColor("_Color", Color.yellow);
break;
case InputActionPhase.Started:
material.SetColor("_Color", Color.green);
break;
case InputActionPhase.Performed://Trigger键按下
material.SetColor("_Color", Color.blue);
break;
case InputActionPhase.Canceled:
material.SetColor("_Color", Color.white);
break;
}
当 Trigger 键处于 InputActionPhase.Performed 阶段时 IsPressed() 方法返回 true
bool isTriggerPressed = rightHandController.activateAction.action.IsPressed();
InputAction中监听按下的方法
WasPressedThisFrame()
在触发按下动作帧中返回true
WasPerformedThisFrame()
在保持按下状态帧中返回true
WasReleasedThisFrame()
在触发释放动作帧中返回true
IsPressed()
只要超过按钮按下阈值就返回true
判断Grip键是否按下
ActionBasedController rightHandController;
//只会在按下那一刻返回true
if (rightHandController.selectAction.action.triggered)
{
Debug.Log("按下手柄上的Grip键");
}
//如果Grip处于按下状态,会一直返回true
if (rightHandController.selectAction.action.IsPressed())
{
Debug.Log("按住手柄上的Grip键");
}
用 triggered 属性判断按下动作,用 IsPressed() 判断是否持续按住不放。
activateAction.action.IsPressed() 与 activateActionValue.action.IsPressed() 没有区别。
按键枚举
namespace UnityEngine.XR.Interaction.Toolkit
{
public static class InputHelpers
{
public enum Button
{
None = 0,
MenuButton = 1,//菜单键
Trigger = 2,//扳机键
Grip = 3,//抓取键
TriggerButton = 4,//扳机键
TriggerPressed = 4,//扳机键
GripButton = 5,//抓取键
GripPressed = 5,//抓取键
PrimaryButton = 6,//右手A键按下 或 左手X键按下
PrimaryTouch = 7,//右手A键触摸 或 左手X键触摸
SecondaryButton = 8,//右手B键按下 或 左手Y键按下
SecondaryTouch = 9,//右手B键触摸 或 左手Y键触摸
Primary2DAxisTouch = 10,//右手摇杆触摸
Primary2DAxisClick = 11,//右手摇杆Click
Secondary2DAxisTouch = 12,//左手摇杆触摸
Secondary2DAxisClick = 13,//左手摇杆Click
PrimaryAxis2DUp = 14,//右手摇杆向上
PrimaryAxis2DDown = 15,//右手摇杆向下
PrimaryAxis2DLeft = 16,//右手摇杆向左
PrimaryAxis2DRight = 17,//右手摇杆向右
SecondaryAxis2DUp = 18,//左手摇杆向上
SecondaryAxis2DDown = 19,//左手摇杆向下
SecondaryAxis2DLeft = 20,//左手摇杆向左
SecondaryAxis2DRight = 21//左手摇杆向右
}
}
}
注意事项
1. 存在两个InputDevice类,分别是 UnityEngine.XR.InputDevice 和 UnityEngine.InputSystem.InputDevice; 建议定义两个别名:
using XRInputDevice = UnityEngine.XR.InputDevice;
using ISInputDevice = UnityEngine.InputSystem.InputDevice;
判断扳机键(Trigger)是否按下
//检测右边手柄扳机键是否按下
XRInputDevice rightHandDevice = InputDevices.GetDeviceAtXRNode(XRNode.RightHand);
if (rightHandDevice.isValid)
{
bool isRightTriggerDown;
//如果一直按住,将持续返回true
rightHandDevice.TryGetFeatureValue(PXR_Usages.triggerTouch, out isRightTriggerDown);
}
判断扳机键(Trigger)是否按下 (推荐)
UnityEngine.XR.Interaction.Toolkit.InputHelpers 类为 UnityEngine.XR.InputDevice 提供了扩展方法:
public static bool IsPressed(this InputDevice device, Button button, out bool isPressed, float pressThreshold = -1);
//检测右边手柄扳机键是否按下
XRInputDevice rightHandDevice = InputDevices.GetDeviceAtXRNode(XRNode.RightHand);
if (rightHandDevice.isValid)
{
bool isPressed;
//如果一直按住,将持续返回true
//经测试:Button.Trigger、Button.TriggerButton、Button.TriggerPressed 3种效果等同
rightHandDevice.IsPressed(InputHelpers.Button.TriggerPressed, out isPressed);
}
模型监听扳机键(Trigger)是否按下
using UnityEngine;
using UnityEngine.XR.Interaction.Toolkit;
public class Test : MonoBehaviour
{
public ActionBasedController rightHandController;
public XRSimpleInteractable interactable;
private void Update()
{
//判断手柄射线是否指向模型
if (interactable.isHovered)
{
//判断是否按下Trigger键
//只会在按下那一刻返回true
if (rightHandController.activateAction.action.triggered)
{
Debug.Log("按下手柄上的Trigger键");
}
}
}
}
using System;
using UnityEngine;
using UnityEngine.Events;
using UnityEngine.Serialization;
using UnityEngine.XR.Interaction.Toolkit;
/// <summary>
/// 挂在模型上,用来监听手柄板机键(Trigger)是否按下
/// </summary>
[RequireComponent(typeof(XRSimpleInteractable))]
public class XRTriggeredEvent : MonoBehaviour
{
public ActionBasedController leftHandController;
public ActionBasedController rightHandController;
public XRSimpleInteractable interactable;
[Serializable]
public class OnTriggeredEvent : UnityEvent { }
[FormerlySerializedAs("triggered")]
[SerializeField]
private OnTriggeredEvent m_OnTriggered = new OnTriggeredEvent();
private void Update()
{
if (interactable.isHovered)
{
bool leftTriggered = false;
bool rightTriggered = false;
if (leftHandController != null)
{
leftTriggered = leftHandController.activateAction.action.triggered;
}
if (rightHandController != null)
{
rightTriggered = rightHandController.activateAction.action.triggered;
}
if (leftTriggered || rightTriggered)
{
//在模型上按下Trigger键(左手或右手)
m_OnTriggered?.Invoke();
}
}
}
}
获取摇杆值
//获取右手摇杆值
XRInputDevice rightHandDevice = InputDevices.GetDeviceAtXRNode(XRNode.RightHand);
if (rightHandDevice.isValid)
{
//取值范围 [-1,1]
//摇杆推到最上面时返回1,推到最下面时返回-1
float singleValue;
rightHandDevice.TryReadSingleValue(InputHelpers.Button.PrimaryAxis2DUp, out singleValue);
}
XRController
建议使用ActionBasedController代替XRController。与ActionBasedController相比,此行为不需要那么多的初始设置,但是输入处理的可定制性较低,并且XRDeviceSimulator不能用于驱动此行为。
创建 UI Canvas
XR UI输入模块(XR UI Input Module)
设置要使用的输入处理系统
[Edit]->Project Settings->Player->Active Input Handling
XR UI Input Module 是与 Event System 关联所必须的组件。 XR UI Input Module 与 XR Ray Interactor(XR射线交互器)协同工作,以确保正确处理与UI的XRI交互。 它还处理来自其他非XR源(如游戏板或鼠标)的输入(如果配置为这样做)。
Enable Builtin Actions As Fallback
如果勾选,这将允许默认的鼠标、游戏板或操纵杆操作在当前输入系统设备配置的情况下驱动UI。
标签: Unity3d
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
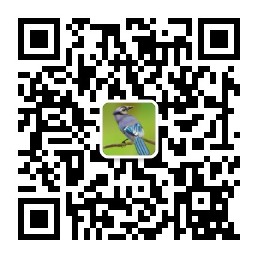