抽象数据类型(ADT)——队列
作者:追风剑情 发布于:2020-4-22 10:34 分类:C
示例:实现一个队列
queue.h
- //#pragma once
- #ifndef _QUEUE_H_
- #define _QUEUE_H_
- #include <stdbool.h>
- typedef int Item;
- #define MAXQUEUE 10
- typedef struct node
- {
- Item item;
- struct node * next;
- } Node;
- typedef struct queue
- {
- Node * front; /* 指向队列首项的指针 */
- Node * rear; /* 指向队列尾项的指针 */
- int items; /* 队列中的项数 */
- } Queue;
- /* 操作: 初始化队列 */
- /* 前提条件: pq指向一个队列 */
- /* 后置条件: 队列被初始化 */
- void InitializeQueue(Queue * pq);
- /* 操作: 检查队列是否已满 */
- /* 前提条件: pq指向之前被初始化的队列 */
- /* 后置条件: 如果队列已满则返回true,否则返回false */
- bool QueueIsFull(const Queue * pq);
- /* 操作: 检查队列是否为空 */
- /* 前提条件: pq指向之前被初始化的队列 */
- /* 后置条件: 如果队列为空则返回true,否则返回false */
- bool QueueIsEmpty(const Queue * pq);
- /* 操作: 确定队列中的项数 */
- /* 前提条件: pq指向之前被初始化的队列 */
- /* 后置条件: 返回队列中的项数 */
- int QueueItemCount(const Queue * pq);
- /* 操作: 在队列末尾添加项 */
- /* 前提条件: pq指向之前被初始化的队列 */
- /* item是要被添加在队列末尾的项 */
- /* 后置条件: 如果队列不为空,item将被添加在队列的末尾*/
- /* 该函数返回true;否则,队列不变,该函数返回false*/
- bool EnQueue(Item item, Queue * pq);
- /* 操作: 从队列的开头删除项 */
- /* 前提条件: pq指向之前被初始化的队列 */
- /* 后置条件: 如果队列不为空,队列首端的item将被拷贝到*pitem中 */
- /* 如果该操作使得队列为空,则重置队列为空 */
- /* 如果队列在操作前为空,该函数返回false */
- bool DeQueue(Item * pitem, Queue * pq);
- /* 操作: 清空队列 */
- /* 前提条件: pq指向之前被初始化的队列 */
- /* 后置条件: 队列被清空 */
- void EmptyTheQueue(Queue * pq);
- #endif
queue.c
- #include <stdio.h>
- #include <stdlib.h>
- #include "queue.h"
- /* 局部函数原型 */
- static void CopyToNode(Item item, Node * pn);
- static void CopyToItem(Node *pn, Item * pi);
- /* 初始化队列 */
- void InitializeQueue(Queue * pq)
- {
- pq->front = pq->rear = NULL;
- pq->items = 0;
- }
- /* 检查队列是否已满 */
- bool QueueIsFull(const Queue * pq)
- {
- return pq->items == MAXQUEUE;
- }
- /* 检查队列是否为空 */
- bool QueueIsEmpty(const Queue * pq)
- {
- return pq->items == 0;
- }
- /* 确定队列中的项数 */
- int QueueItemCount(const Queue * pq)
- {
- return pq->items;
- }
- /* 在队列末尾添加项 */
- bool EnQueue(Item item, Queue * pq)
- {
- Node * pnew;
- if (QueueIsFull(pq))
- return false;
- pnew = (Node *)malloc(sizeof(Node));
- if (pnew == NULL)
- {
- fprintf(stderr, "Unable to allocate memory!\n");
- exit(1);
- }
- CopyToNode(item, pnew);
- pnew->next = NULL;
- if (QueueIsEmpty(pq))
- pq->front = pnew; /* 项位于队列首端 */
- else
- pq->rear->next = pnew; /* 链接到队列尾端 */
- pq->rear = pnew; /* 记录队列尾端的位置 */
- pq->items++; /* 队列项数加1 */
- return true;
- }
- /* 从队列的开头删除项 */
- bool DeQueue(Item * pitem, Queue * pq)
- {
- Node * pt;
- if (QueueIsEmpty(pq))
- return false;
- CopyToItem(pq->front, pitem);
- pt = pq->front;
- pq->front = pq->front->next;
- free(pt);
- pq->items--;
- if (pq->items == 0)
- pq->rear = NULL;
- return true;
- }
- /* 清空队列 */
- void EmptyTheQueue(Queue * pq)
- {
- Item dummy;
- while (!QueueIsEmpty(pq))
- DeQueue(&dummy, pq);
- }
- /* 局部函数 */
- static void CopyToNode(Item item, Node * pn)
- {
- pn->item = item;
- }
- static void CopyToItem(Node *pn, Item * pi)
- {
- *pi = pn->item;
- }
main.c
- #define _CRT_SECURE_NO_WARNINGS
- //可以用#ifndef指令,防止多次包含一个文件
- #include <stdio.h>
- //提供malloc()原型
- #include <stdlib.h>
- //提供strcpy()原型
- #include <string.h>
- //提供CHAR_BIT的定义,CHAR_BIT表示每字节的位数
- #include <limits.h>
- //C99定义了bool、true、false
- //如果编译器不支持bool,可以用枚举替代enum bool {false, true};
- #include <stdbool.h>
- #include "queue.h"
- int main(int argc, char* argv[])
- {
- Queue line;
- Item temp;
- char ch;
- InitializeQueue(&line);
- puts("Testing the Queue interface. Type a to add a value, ");
- puts("type d to delete a value, and type q to quit.");
- while ((ch = getchar()) != 'q')
- {
- if (ch != 'a' && ch != 'd') /* 忽略其他输出 */
- continue;
- if (ch == 'a')
- {
- printf("Integer to add: ");
- scanf("%d", &temp);
- if (!QueueIsFull(&line))
- {
- printf("Putting %d into queue\n", temp);
- EnQueue(temp, &line);
- }
- else
- puts("Queue is full!");
- }
- else
- {
- if (QueueIsEmpty(&line))
- puts("Nothing to delete!");
- else
- {
- DeQueue(&temp, &line);
- printf("Removing %d from queue\n", temp);
- }
- }
- printf("%d items in queue\n", QueueItemCount(&line));
- puts("Type a to add, d to delete, q to quit:");
- }
- EmptyTheQueue(&line);
- puts("Bye!");
- system("pause");
- return 0;
- }
标签: C语言
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年3月(4)
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
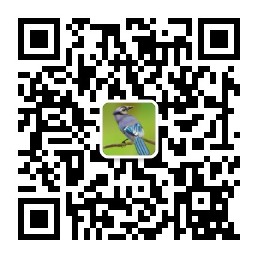