抽象数据类型(ADT)——链表
作者:追风剑情 发布于:2020-4-21 11:23 分类:C
示例:通过链表储存用户输入的影片信息
list.h
//#pragma once //防止多次包含头文件 #ifndef LIST_H_ #define LIST_H_ #include <stdbool.h> /* C99特性 */ /* 特定程序的声明 */ #define TSIZE 45 /* 储存电影名的数组大小 */ struct film { char title[TSIZE]; int rating; }; /* 一般类型定义 */ typedef struct film Item; typedef struct node { Item item; struct node * next; } Node; typedef Node * List; /* List的另一种定义 */ /* typedef struct list { Node * head; //指向链表头的指针 Node * end; //指向链表尾的指针 int size; //链表中的项数 } List; */ /* 函数原型 */ /* 操作: 初始化一个链表 */ /* 前提条件: plist指向一个链表 */ /* 后置条件: 链表初始化为空 */ void InitializeList(List * plist); /* 操作: 确定链表是否为空,plist指向一个已初始化的链表 */ /* 后置条件: 如果链表为空,该函数返回true;否则返回false */ bool ListIsEmpty(const List * plist); /* 操作: 确定链表是否已满,plist指向一个已初始化的链表 */ /* 后置条件: 如果链表已满,该函数返回true;否则返回false */ bool ListIsFull(const List * plist); /* 操作: 确定链表中的项数,plist指向一个已初始化的链表 */ /* 后置条件: 该函数返回链表中的基数 */ unsigned int ListItemCount(const List * plist); /* 操作: 在链表的末尾添加项 */ /* 前提条件: item是一个待添加至链表的项,plist指向一个已初始化的链表 */ /* 后置条件: 如果可以,该函数在链表末尾添加一个项,且返回true,否则返回false */ bool AddItem(Item item, List * plist); /* 操作: 把函数作用于链表中的每一项 */ /* plist指向一个已初始化的链表 */ /* pfun指向一个函数,该函数接受一个Item类型的参数,且无返回值 */ /* 后置条件: pfun指向的函数作用于链表中的每一项一次 */ void Traverse(const List * plist, void(*pfun)(Item item)); /* 操作: 释放已分配的内存(如果有的话) */ /* plist指向一个已初始化的链表 */ void EmptyTheList(List * plist); #endif
list.c
#include <stdio.h> #include <stdlib.h> #include "list.h" /* 局部函数原型 */ static void CopyToNode(Item item, Node * pnode); void InitializeList(List * plist) { *plist = NULL; } /* 如果链表为空,返回true */ bool ListIsEmpty(const List * plist) { if (*plist == NULL) return true; return false; } /* 如果链表已满, 返回true */ bool ListIsFull(const List * plist) { Node * pt; bool full; pt = (Node *)malloc(sizeof(Node)); if (pt == NULL) //分配内存失败 full = true; else full = false; free(pt); return full; } /* 返回节点的数量 */ unsigned int ListItemCount(const List * plist) { unsigned int count = 0; Node * pnode = *plist; /* 设置链表的开始 */ while (pnode != NULL) { ++count; pnode = pnode->next; /* 设置下一个节点 */ } return count; } /* 创建储存项的节点,并将其添加至由plist指向的链表末尾(较慢的实现) */ bool AddItem(Item item, List * plist) { Node * pnew; Node * scan = *plist; pnew = (Node *)malloc(sizeof(Node)); if (pnew == NULL) //分配内存失败 return false; CopyToNode(item, pnew); pnew->next = NULL; if (scan == NULL) //如果链表为空 *plist = pnew; else { while (scan->next != NULL) scan = scan->next; //找到链表的末尾 scan->next = pnew; //把pnew添加到链表的末尾 } return true; } /* 访问每个节点并执行pfun指向的函数 */ void Traverse(const List * plist, void(*pfun)(Item item)) { Node * pnode = *plist; /* 设置链表的开始 */ while (pnode != NULL) { (*pfun)(pnode->item); /* 把函数应用于链表中的项 */ pnode = pnode->next; } } /* 释放由malloc()分配的内存 */ /* 释放链表指针为NULL */ void EmptyTheList(List * plist) { Node * psave; while (*plist != NULL) { psave = (*plist)->next; free(*plist); *plist = psave; } } /* 局部函数 */ /* 把一个项拷贝到节点中 */ static void CopyToNode(Item item, Node * pnode) { pnode->item = item; }
Main.c
#define _CRT_SECURE_NO_WARNINGS //可以用#ifndef指令,防止多次包含一个文件 #include <stdio.h> //提供malloc()原型 #include <stdlib.h> //提供strcpy()原型 #include <string.h> //提供CHAR_BIT的定义,CHAR_BIT表示每字节的位数 #include <limits.h> //C99定义了bool、true、false //如果编译器不支持bool,可以用枚举替代enum bool {false, true}; #include <stdbool.h> #include "list.h" void showmovies(Item item); char* s_gets(char* st, int n); int main(int argc, char* argv[]) { List movies; Item temp; /* 初始化 */ InitializeList(&movies); if (ListIsFull(&movies)) { fprintf(stderr, "No memory available! Bye!\n"); exit(1); } /* 获取用户输入并储存 */ puts("Enter first movie title:"); while (s_gets(temp.title, TSIZE) != NULL && temp.title[0] != '\0') { puts("Enter your rating<0-10>:"); scanf("%d", &temp.rating); while (getchar() != '\n') continue; if (AddItem(temp, &movies) == false) { fprintf(stderr, "Problem allocating memory\n"); break; } if (ListIsFull(&movies)) { puts("The list is now full."); break; } puts("Enter next movie title (empty line to stop):"); } /* 显示 */ if (ListIsEmpty(&movies)) printf("No data entered."); else { printf("Here is the movie list:\n"); Traverse(&movies, showmovies); } printf("You entered %d movies.\n", ListItemCount(&movies)); /* 清理 */ EmptyTheList(&movies); printf("Bye!\n"); system("pause"); return 0; } void showmovies(Item item) { printf("Movie: %s Rating: %d\n", item.title, item.rating); } // 自己实现读取函数 char* s_gets(char* st, int n) { char* ret_val; int i = 0; ret_val = fgets(st, n, stdin); if (ret_val) //即,ret_val != NULL { while (st[i] != '\n' && st[i] != '\0') i++; if (st[i] == '\n') st[i] = '\0'; else while (getchar() != '\n') continue; } return ret_val; }
标签: C语言
« 抽象数据类型(ADT)——队列
|
NGUI分割图集»
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年10月(4)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
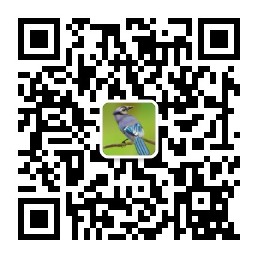