函数和指针
作者:追风剑情 发布于:2020-3-16 14:43 分类:C
示例
#define _CRT_SECURE_NO_WARNINGS #include <stdio.h> #include <stdlib.h> #include <stdbool.h> //C99特性 #include <string.h> #include <math.h> #include <ctype.h> //函数指针指向函数代码起始处的内存地址 //要注意运算符*的优先级 //void (*pf)(char *); //pf是一个指向函数的指针 //void *pf(char *); //pf是一个返回字符指针的函数 /* 提示: 要声明一个指向特定类型函数的指针,可以先声明一个该类型的函数,然后把函数名替换成(*pf) 形式的表达式。然后,pf就成为指向该类型函数的指针。 */ #define LEN 81 char* s_gets(char *st, int n); char showmenu(void); void eatline(void); // 读取至行末尾 void show(void(*fp)(char *), char * str); void ToUpper(char *); // 把字符串转换为大写 void ToLower(char *); // 把字符串转换为小写 void Transpose(char *); // 大小写转置 void Dummy(char *); // 不更改字符串 //typedef void (*V_FP_CHARP) (char *); int main(int argc, char* argv[]) { char line[LEN]; char copy[LEN]; char choice; // 声明一个函数指针,被指向的函数接受char *类型的参数,无返回值 void (*pfun) (char *); //或者这样声明 //V_FP_CHARP pfun; pfun = Dummy; puts("Enter a string (empty line to quit):"); while (s_gets(line, LEN) != NULL && line[0] != '\0') { while ((choice = showmenu()) != 'n') { switch (choice) { //函数名代表函数的地址 //注意:只能把类型匹配的函数地址赋给pfun case 'u': pfun = ToUpper; break; case 'l': pfun = ToLower; break; case 't': pfun = Transpose; break; case 'o': pfun = Dummy; break; } strcpy(copy, line); // 为show()函数拷贝一份 show(pfun, copy); // 根据用户的选择,使用选定的函数 } puts("Enter a string (empty line to quit):"); } puts("Bye!"); system("pause"); return 0; } char showmenu(void) { char ans; puts("Enter menu choice:"); puts("u) uppercase l) lowercase"); puts("t) transposed case o) original case"); puts("n) next string"); ans = getchar(); // 获取用户输入 ans = tolower(ans); // 转换为小写 eatline(); //清理输入 //判断输入的字符是否包含在ulton字符串中 while (strchr("ulton", ans) == NULL) { puts("Please enter a u, l, t, o, or n:"); ans = tolower(getchar()); eatline(); } return ans; } void eatline(void) { while (getchar() != '\n') continue; } void ToUpper(char* str) { while (*str) { *str = toupper(*str); str++; } } void ToLower(char* str) { while (*str) { *str = tolower(*str); str++; } } void Transpose(char* str) { while (*str) { if (islower(*str)) *str = toupper(*str); else if (isupper(*str)) *str = tolower(*str); str++; } } void Dummy(char* str) { // 不改变字符串 } //void show(V_FP_CHARP fp, char *) void show(void(*fp)(char*), char* str) { //由于历史原因ANSI C认为下面两种函数调用方式等价 (*fp)(str); // 把用户选定的函数作用于str //fp(str); //与(*fp)(str)写法等效 puts(str); // 显示结果 } // 自己实现读取函数 char* s_gets(char* st, int n) { char* ret_val; int i = 0; ret_val = fgets(st, n, stdin); if (ret_val) //即,ret_val != NULL { while (st[i] != '\n' && st[i] != '\0') i++; if (st[i] == '\n') st[i] = '\0'; else while (getchar() != '\n') continue; } return ret_val; }
运行测试
标签: C语言
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年11月(3)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
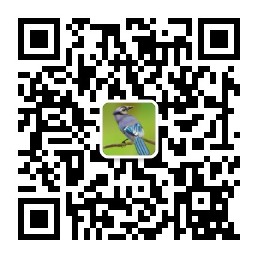