C语言—函数
作者:追风剑情 发布于:2019-9-16 20:47 分类:C
示例一:一个简单函数
- //Visual Studio中加上这句才可以使用scanf()
- //否则只能使用scanf_s()
- #define _CRT_SECURE_NO_WARNINGS
- #include <stdio.h>
- #include <stdbool.h>
- #define NAME "GIGATHINK, INC."
- #define ADDRESS "101 Megabuck Plaza"
- #define PLACE "Megapolis, CA 94904"
- #define WIDTH 40
- void starbar(void); /* ANSI C风格函数原型 */
- //void starbar(); /* 对于不识别ANSI C风格的编译器这样声明 */
- //一些老的编译器甚至连void都识别不了
- //argc: 参数个数 argv[]: 参数数组
- int main(int argc, char *argv[])
- {
- // 也可以在函数里面声明
- //void starbar(void);
- starbar();
- printf("%s\n", NAME);
- printf("%s\n", ADDRESS);
- printf("%s\n", PLACE);
- starbar(); /* 调用函数 */
- system("pause");
- return 0;
- }
- void starbar(void) /* 定义函数 */
- {
- int count;
- for (count = 1; count <= WIDTH; count++)
- putchar('*');
- putchar('\n');
- }
示例二:对示例一进行改进
- //Visual Studio中加上这句才可以使用scanf()
- //否则只能使用scanf_s()
- #define _CRT_SECURE_NO_WARNINGS
- #include <stdio.h>
- #include <stdbool.h>
- #define NAME "GIGATHINK, INC."
- #define ADDRESS "101 Megabuck Plaza"
- #define PLACE "Megapolis, CA 94904"
- #define WIDTH 40
- #define SPACE ' '
- // 推荐的声明方式
- void show_n_char(char ch, int num);
- // 推荐的声明方式,根据个人喜好声明函数时也可以不写变量名
- //void show_n_char(char, int);
- // 也可以不声明参数(已废弃)
- //void show_n_char();
- // ANSI C之前的声明形式(已废弃)
- //void show_n_char(ch, num)
- //char ch;
- //int num;
- //argc: 参数个数 argv[]: 参数数组
- int main(int argc, char *argv[])
- {
- int spaces;
- show_n_char('*', WIDTH);
- putchar('\n');
- show_n_char(SPACE, 12);
- printf("%s\n", NAME);
- spaces = (WIDTH - strlen(ADDRESS)) / 2;
- show_n_char(SPACE, spaces);
- printf("%s\n", ADDRESS);
- show_n_char(SPACE, (WIDTH - strlen(PLACE)) / 2);
- printf("%s\n", PLACE);
- show_n_char('*', WIDTH);
- putchar('\n');
- system("pause");
- return 0;
- }
- void show_n_char(char ch, int num)
- {
- int count;
- for (count = 1; count <= num; count++)
- putchar(ch);
- }
示例:函数返回值
- //Visual Studio中加上这句才可以使用scanf()
- //否则只能使用scanf_s()
- #define _CRT_SECURE_NO_WARNINGS
- #include <stdio.h>
- #include <stdbool.h>
- int imin(int, int);
- // ANSI C允许使用部分原型(实用于函数参数不固定的情况)。
- // 如: int printf(const char *, ...);
- //argc: 参数个数 argv[]: 参数数组
- int main(int argc, char *argv[])
- {
- int evil1, evil2;
- printf("Enter a pair of integers (q to quit):\n");
- while (scanf("%d %d", &evil1, &evil2) == 2)
- {
- printf("The lesser of %d and %d is %d.\n",
- evil1, evil2, imin(evil1, evil2));
- printf("Enter a pair of integers (q to quit):\n");
- }
- printf("Bye.\n");
- system("pause");
- return 0;
- }
- // 如果把函数定义放在main()之前,则可以省略函数声明。
- int imin(int n, int m)
- {
- return (n < m) ? n : m;
- }
示例:递归
- //Visual Studio中加上这句才可以使用scanf()
- //否则只能使用scanf_s()
- #define _CRT_SECURE_NO_WARNINGS
- #include <stdio.h>
- #include <stdbool.h>
- void up_and_down(int);
- //argc: 参数个数 argv[]: 参数数组
- int main(int argc, char *argv[])
- {
- up_and_down(1);
- system("pause");
- return 0;
- }
- void up_and_down(int n)
- {
- printf("Level %d: n location %p\n", n, &n);
- if (n < 4)
- up_and_down(n + 1);
- printf("LEVEL %d: n location %p\n", n, &n);
- }
示例:尾递归
运行测试
- //Visual Studio中加上这句才可以使用scanf()
- //否则只能使用scanf_s()
- #define _CRT_SECURE_NO_WARNINGS
- #include <stdio.h>
- #include <stdbool.h>
- long fact(int n);
- long rfact(int n);
- //argc: 参数个数 argv[]: 参数数组
- int main(int argc, char *argv[])
- {
- int num;
- printf("This program calculates factorials.\n");
- printf("Enter a value in the range 0-12 (q to quit):\n");
- while (scanf("%d", &num) == 1)
- {
- if (num < 0)
- printf("No negative numbers, please.\n");
- else if (num > 12)
- printf("Keep input under 13.\n");
- else
- {
- printf("loop: %d factorial = %ld\n",
- num, fact(num));
- printf("recursion: %d factorial = %ld\n",
- num, rfact(num));
- }
- printf("Enter a value in the range 0-12 (q to quit):\n");
- }
- printf("Bye.\n");
- system("pause");
- return 0;
- }
- // 使用循环计算
- long fact(int n)
- {
- long ans;
- for (ans = 1; n > 1; n--)
- ans *= n;
- return ans;
- }
- // 使用递归计算
- long rfact(int n)
- {
- long ans;
- if (n > 0)
- ans = n * rfact(n - 1);
- else
- ans = 1;
- return ans;
- }
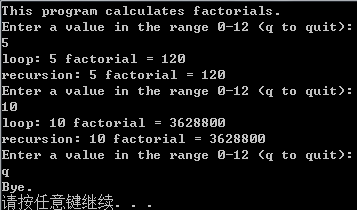
示例:递归和倒序计算
运行测试
- //Visual Studio中加上这句才可以使用scanf()
- //否则只能使用scanf_s()
- #define _CRT_SECURE_NO_WARNINGS
- #include <stdio.h>
- #include <stdbool.h>
- void to_binary(unsigned long n);
- //argc: 参数个数 argv[]: 参数数组
- int main(int argc, char *argv[])
- {
- unsigned long number;
- printf("Enter an integer (q to quit):\n");
- while (scanf("%lu", &number) == 1)
- {
- printf("Binary equivalent: ");
- to_binary(number);
- putchar('\n');
- printf("Enter an integer (q to quit):\n");
- }
- printf("Done.\n");
- system("pause");
- return 0;
- }
- // 使用递归将十进制数用二进制表示
- void to_binary(unsigned long n)
- {
- int r;
- r = n % 2;
- if (n >= 2)
- to_binary(n / 2);
- putchar(r == 0 ? '0' : '1');
- }
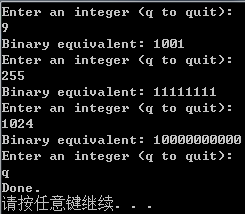
标签: C语言
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年3月(4)
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
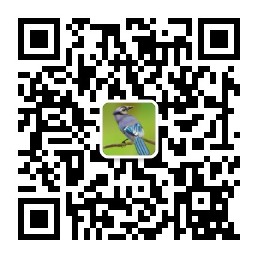