图的广度优先搜索
作者:追风剑情 发布于:2014-8-24 12:43 分类:Algorithms
程序语言 C++开发工具 Visual Studio2010
Graph.h文件
- #define MAXVEX 5 //图中最大顶点数
- /////////////////////////////////////////////////////////////////////
- //
- // 定义邻接表数据结构
- //
- /////////////////////////////////////////////////////////////////////
- //定义顶点类型为10个字符的字符数组
- typedef char VertexType[10];
- typedef struct edgenode
- {
- int adjvex; //邻接点序号
- int value; //边的权值
- struct edgenode *next; //下一条边的顶点
- } ArcNode; //每个顶点建立的单链表中结点的类型
- typedef struct vexnode
- {
- VertexType data; //顶点信息
- ArcNode *firstarc; //指向第一条边结点
- } VHeadNode; //单链表的头结点类型
- typedef struct
- {
- int n,e; //n为实际顶点数,e为实际边数
- VHeadNode adjlist[MAXVEX]; //单链表头结点数组
- } AdjList; //图的邻接表类型
- /////////////////////////////////////////////////////////////////////
- //
- // 定义邻接矩阵数据结构
- //
- /////////////////////////////////////////////////////////////////////
- typedef struct vertex
- {
- int adjvex; /*顶点编号*/
- VertexType data;/*顶点信息*/
- } VType; /*顶点类型*/
- typedef struct graph
- {
- int n,e; /*n为实际顶点数,e为实际边数*/
- VType vexs[MAXVEX]; /*顶点集合*/
- int edges[MAXVEX][MAXVEX]; /*边的集合*/
- } AdjMatix; /*图的邻接矩阵类型*/
GSearch.cpp文件
- #include <stdlib.h>
- #include <stdio.h>
- #include <string.h>
- #include "Graph.h"
- //将邻接矩阵g转换成邻接表G*
- void MatToList(AdjMatix g, AdjList *&G)
- {
- int i,j;
- ArcNode *p;
- G = (AdjList *)malloc(sizeof(AdjList));
- for(i=0; i<g.n; i++) //给邻接表中所有头结点的指针域置初值
- {
- G->adjlist[i].firstarc = NULL;
- strcpy(G->adjlist[i].data, g.vexs[i].data);
- }
- //检查邻接矩阵中每个元素
- for(i=0; i<g.n; i++)
- {
- for(j=g.n - 1; j>=0; j--)
- {
- if(g.edges[i][j] != 0){
- //创建一个结点p
- p = (ArcNode *)malloc(sizeof(ArcNode));
- p->value = g.edges[i][j];
- p->adjvex = j;
- //将p插入到链表之首
- p->next = G->adjlist[i].firstarc;
- G->adjlist[i].firstarc = p;
- }
- }
- }
- G->n = g.n;
- G->e = g.e;
- }
- void DispAdjList(AdjList *G)
- {
- int i;
- ArcNode *p;
- printf("图的邻接表表示如下:\n");
- for(i=0; i<G->n; i++)
- {
- printf("[%d, %3s]=>", i, G->adjlist[i].data);
- p = G->adjlist[i].firstarc;
- while(p != NULL)
- {
- printf("(%d, %d)->", p->adjvex, p->value);
- p = p->next;
- }
- printf("NULL\n");
- }
- }
- //对邻接表G从顶点vi开始进行广度优先遍历
- void BFS(AdjList *G, int vi)
- {
- int i,v,visited[MAXVEX];
- int Qu[MAXVEX], front=0, rear=0; //循环队列
- ArcNode *p;
- for (i=0; i<G->n; i++) //给visited数组置初值0
- visited[i] = 0;
- printf("%d ", vi); //访问初始顶点
- visited[vi] = 1; //置已访问标识
- rear = (rear=1) % MAXVEX; //循环移动队尾指针
- Qu[rear] = vi; //初始顶点进队列
- while (front != rear) //队列不为空时循环
- {
- front = (front + 1) % MAXVEX;
- v = Qu[front]; //顶点v出队
- p = G->adjlist[v].firstarc;//找v的第一个邻接点
- while (p != NULL) //找v的所有邻接点
- {
- if (visited[p->adjvex] == 0) //未访问过则访问它
- {
- visited[p->adjvex] = 1; //置已访问标识
- printf("%d ", p->adjvex);//访问该点并使其入队列
- rear = (rear + 1) % MAXVEX; //循环移动队尾指针
- Qu[rear] = p->adjvex;//顶点p->adjvex进队
- }
- p = p->next; //找v的下一个邻接点
- }
- }
- }
- void main()
- {
- int i,j;
- AdjMatix g;
- AdjList *G;
- int a[5][5] = {{0,1,0,1,0},{1,0,1,0,0},{0,1,0,1,1},
- {1,0,1,0,1},{0,0,1,1,0}};
- char *vname[MAXVEX] = {"a", "b", "c", "d", "e"};
- g.n = 5;
- g.e = 12;//建立无向图,每1条无向边算2条有向边
- for(i=0; i<g.n; i++)
- strcpy(g.vexs[i].data, vname[i]);
- for(i=0; i<g.n; i++)
- for(j=0; j<g.n; j++)
- g.edges[i][j] = a[i][j];
- MatToList(g, G);
- DispAdjList(G);
- printf("从顶点0的广度优先遍历序列:\n");
- BFS(G, 0);
- printf("\n");
- system("pause");
- }
工程结构
运行结果
标签: Algorithms
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年3月(4)
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
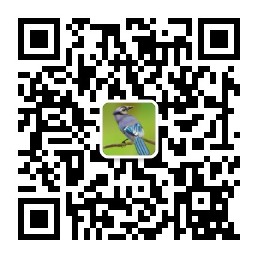