单字符I/O: getchar()和putchar()
作者:追风剑情 发布于:2019-9-5 21:27 分类:C
//Visual Studio中加上这句才可以使用scanf() //否则只能使用scanf_s() #define _CRT_SECURE_NO_WARNINGS #include <stdio.h> #include <stdbool.h> // 验证输入是一个整数 long get_long(void); // 验证范围的上下限是否有效 bool bad_limits(long begin, long end, long low, long high); // 计算a~b之间的整数平方和 double sum_squares(long a, long b); //argc: 参数个数 argv[]: 参数数组 int main(int argc, char *argv[]) { const long MIN = -10000000L; // 范围的下限 const long MAX = +10000000L; // 范围的上限 long start; // 用户指定的范围最小值 long stop; // 用户指定的范围最大值 double answer; printf("This program computes the sum of the squares of " "integers in a range.\nThe lower bound should not " "be less than -10000000 and \nthe upper bound " "should not be more than +10000000.\nEnter the " "limits (enter 0 for both limits to quit):\n" "lower limit: "); start = get_long(); printf("upper limit: "); stop = get_long(); while (start != 0 || stop != 0) { if (bad_limits(start, stop, MIN, MAX)) printf("Please try again.\n"); else { answer = sum_squares(start, stop); printf("The sum of the squares of the integers "); printf("from %ld to %ld is %g\n", start, stop, answer); } printf("Enter the limits (enter 0 for both " "limits to quit):\n"); printf("lower limit: "); start = get_long(); printf("upper limit: "); stop = get_long(); } printf("Done.\n"); system("pause"); return 0; } long get_long(void) { long input; char ch; //scanf返回正确读入的个数 while (scanf("%ld", &input) != 1) { while ((ch = getchar()) != '\n') putchar(ch); //处理错误输入 printf(" is not an integer.\nPlease enter an"); printf("integer value, such as 25, -178, or 3: "); } return input; } double sum_squares(long a, long b) { double total = 0; long i; for (i = a; i <= b; i++) total += (double)i * (double)i; return total; } bool bad_limits(long begin, long end, long low, long high) { bool not_good = false; if (begin > end) { printf("%ld isn't smaller than %ld.\n", begin, end); not_good = true; } if (begin < low || end < low) { printf("Values must be %ld or greater.\n", low); not_good = true; } if (begin > high || end > high) { printf("Values must be %ld or less.\n", high); not_good = true; } return not_good; }
getchar()和putchar()每次只处理一个字符。
示例:获取从键盘输入的字符,并把这些字符发送到屏幕
//Visual Studio中加上这句才可以使用scanf() //否则只能使用scanf_s() #define _CRT_SECURE_NO_WARNINGS #include <stdio.h> //argc: 参数个数 argv[]: 参数数组 int main(int argc, char *argv[]) { char ch = 'a'; //循环读取键盘输入的字符,并存入缓冲区 //当按下回车后输出到屏幕 while ((ch = getchar()) != '#') putchar(ch);//存入缓冲区,按回车后输出到屏幕 system("pause"); return 0; }运行测试
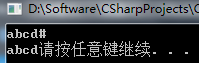
缓冲区
缓冲分两类:
完全缓冲I/O和行缓冲I/O。完全缓冲输入指的是当缓冲区被填满时才刷新缓冲区(内容被发至目的地),通常出现在文件输入中。缓冲区的大小取决于系统,常见的大小是512字节和4096字节。行缓冲I/O指的是在出现换行符时刷新缓冲区。键盘输入通常是行缓冲输入,所以在按下Enter键后才刷新缓冲区。是否能进行无缓冲输入取决于计算机系统。
示例:文件结尾符EOF(End Of File)
//Visual Studio中加上这句才可以使用scanf() //否则只能使用scanf_s() #define _CRT_SECURE_NO_WARNINGS #include <stdio.h> //argc: 参数个数 argv[]: 参数数组 int main(int argc, char *argv[]) { //EOF(End Of File)在stdio.h中被定义#define EOF (-1) //MS-DOS中会将行首的Ctrl+Z识为结尾符EOF //UNIX和Linux中,会将行首的Ctrl+D识别为结尾符 //还有一些其他系统会将任意位置的Ctrl+Z识别为结尾符 int ch; while ((ch = getchar()) != EOF) { putchar(ch); } system("pause"); return 0; }运行测试
重定向输入输出
示例:在CMD中运行上例生成的exe文件
< 符号为重定向输入;> 符号为重定向输出
重定向运算符不区别先后,以下两句都是正确的:
CCC.exe < in.txt > out.txt
CCC.exe > out.txt < in.txt
注意: 一些系统要求重定向运算符左侧要有一个空格,右侧没有空格。而其他系统(如,UNIX)允许在重定位运算符两侧有空格或没空格。
示例:输入验证
//Visual Studio中加上这句才可以使用scanf() //否则只能使用scanf_s() #define _CRT_SECURE_NO_WARNINGS #include <stdio.h> #include <stdbool.h> // 验证输入是一个整数 long get_long(void); // 验证范围的上下限是否有效 bool bad_limits(long begin, long end, long low, long high); // 计算a~b之间的整数平方和 double sum_squares(long a, long b); //argc: 参数个数 argv[]: 参数数组 int main(int argc, char *argv[]) { const long MIN = -10000000L; // 范围的下限 const long MAX = +10000000L; // 范围的上限 long start; // 用户指定的范围最小值 long stop; // 用户指定的范围最大值 double answer; printf("This program computes the sum of the squares of " "integers in a range.\nThe lower bound should not " "be less than -10000000 and \nthe upper bound " "should not be more than +10000000.\nEnter the " "limits (enter 0 for both limits to quit):\n" "lower limit: "); start = get_long(); printf("upper limit: "); stop = get_long(); while (start != 0 || stop != 0) { if (bad_limits(start, stop, MIN, MAX)) printf("Please try again.\n"); else { answer = sum_squares(start, stop); printf("The sum of the squares of the integers "); printf("from %ld to %ld is %g\n", start, stop, answer); } printf("Enter the limits (enter 0 for both " "limits to quit):\n"); printf("lower limit: "); start = get_long(); printf("upper limit: "); stop = get_long(); } printf("Done.\n"); system("pause"); return 0; } long get_long(void) { long input; char ch; //scanf返回正确读入的个数 while (scanf("%ld", &input) != 1) { while ((ch = getchar()) != '\n') putchar(ch); //处理错误输入 printf(" is not an integer.\nPlease enter an"); printf("integer value, such as 25, -178, or 3: "); } return input; } double sum_squares(long a, long b) { double total = 0; long i; for (i = a; i <= b; i++) total += (double)i * (double)i; return total; } bool bad_limits(long begin, long end, long low, long high) { bool not_good = false; if (begin > end) { printf("%ld isn't smaller than %ld.\n", begin, end); not_good = true; } if (begin < low || end < low) { printf("Values must be %ld or greater.\n", low); not_good = true; } if (begin > high || end > high) { printf("Values must be %ld or less.\n", high); not_good = true; } return not_good; }运行测试
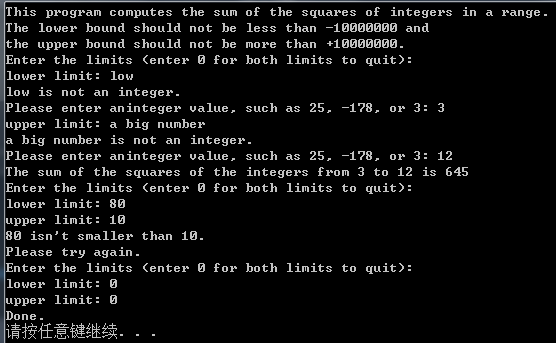
标签: C语言
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年11月(3)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
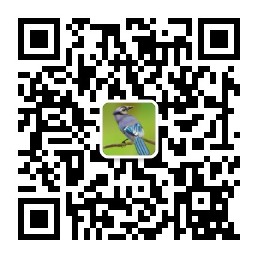