触控旋转及缩放模型
作者:追风剑情 发布于:2019-8-30 17:06 分类:LayaAir
示例:直接在官方示例上改的
import { ui } from "./../ui/layaMaxUI"; /** * 本示例采用非脚本的方式实现,而使用继承页面基类,实现页面逻辑。在IDE里面设置场景的Runtime属性即可和场景进行关联 * 相比脚本方式,继承式页面类,可以直接使用页面定义的属性(通过IDE内var属性定义),比如this.tipLbll,this.scoreLbl,具有代码提示效果 * 建议:如果是页面级的逻辑,需要频繁访问页面内多个元素,使用继承式写法,如果是独立小模块,功能单一,建议用脚本方式实现,比如子弹脚本。 */ export default class GameUI extends ui.test.TestSceneUI { // 触控操作的对象 private box: Laya.MeshSprite3D; // 当前加载的模型 private cabin:Laya.MeshSprite3D; // 一个日志文本框 private logTextArea:Laya.TextArea; private _scene: Laya.Scene3D; constructor() { super(); //初始化引擎 Laya3D.init(0, 0); //适配模式 Laya.stage.fullScreenEnabled = true; Laya.stage.scaleMode = Laya.Stage.SCALE_FULL; Laya.stage.screenMode = Laya.Stage.SCREEN_VERTICAL; Laya.stage.frameRate = Laya.Stage.FRAME_SLOW; //开启统计信息 //Laya.Stat.show(Laya.Browser.clientWidth - 180); //添加3D场景 this._scene = Laya.stage.addChild(new Laya.Scene3D()) as Laya.Scene3D; //添加照相机 var camera: Laya.Camera = (this._scene.addChild(new Laya.Camera(0, 0.1, 100))) as Laya.Camera; camera.transform.translate(new Laya.Vector3(0, 2, 3)); camera.transform.rotate(new Laya.Vector3(-30, 0, 0), true, false); //添加方向光 var directionLight: Laya.DirectionLight = this._scene.addChild(new Laya.DirectionLight()) as Laya.DirectionLight; directionLight.color = new Laya.Vector3(0.6, 0.6, 0.6); directionLight.transform.worldMatrix.setForward(new Laya.Vector3(1, -1, 0)); //添加自定义模型 // this.box = this._scene.addChild(new Laya.MeshSprite3D(Laya.PrimitiveMesh.createBox(1, 1, 1))) as Laya.MeshSprite3D; // this.box.transform.rotate(new Laya.Vector3(0, 45, 0), false, false); // //this.box.transform.localScale = new Laya.Vector3(1, 1, 1); // var material: Laya.BlinnPhongMaterial = new Laya.BlinnPhongMaterial(); // material.albedoColor = new Laya.Vector4(1, 1, 0, 1);//反射光颜色(偏黄) // Laya.Texture2D.load("res/layabox.png", Laya.Handler.create(null, function(tex:Laya.Texture2D) { // material.albedoTexture = tex; // })); // this.box.meshRenderer.material = material; //资源url var meshUrl = "res/models/FurnishedCabin/Meshes/Sofa-Sofa.lm"; var materialUrl = "res/models/FurnishedCabin/Meshes/Materials/Chairs_MAT.lmat"; //加载Mesh Laya.Mesh.load(meshUrl, Laya.Handler.create(this, function(mesh){ this.cabin = new Laya.MeshSprite3D(mesh); this._scene.addChild(this.cabin); //加载Material Laya.PBRStandardMaterial.load(materialUrl, Laya.Handler.create(this, function(material) { this.cabin.meshRenderer.material = material; }, null, true)); this.box = this.cabin; }, null, true)); Laya.MouseManager.multiTouchEnabled = true; Laya.stage.on(Laya.Event.MOUSE_DOWN,this, this.onMouseDown); Laya.stage.on(Laya.Event.MOUSE_UP,this, this.onMouseUp); this.logTextArea = new Laya.TextArea(""); this.logTextArea.mouseEnabled = false; this.logTextArea.text = ""; this.logTextArea.multiline = true; this.logTextArea.color = "#00FF00"; this.logTextArea.fontSize = 50; this.logTextArea.width = 1000; this.logTextArea.height = Laya.Browser.clientHeight * 3; Laya.stage.addChild(this.logTextArea); //每帧驱动onLoop()方法 Laya.timer.frameLoop(1, this, this.onLoop, null, false); } // 旋转控制开关 private _rotationEnabled = false; // 旋转因子,用来调节旋转灵敏度 private _factor = 0.3; // 旋转角度 private _rotation = new Laya.Vector3(0, 0, 0); // 记录按下时的坐标 private _downX = -1; private _downY = -1; // 缩放控制开关 private _scaleEnabled = false; // 缩放因子,用来调节缩放灵敏度s private _scaleFactor = 0.000001; // 记录上一帧两触控点距离 private _touchDistance = 0; // 记录缩放值 private _scale: Laya.Vector3 = new Laya.Vector3(1, 1, 1); private _twoFirst = true; private onMouseDown(e:Laya.Event):void { var touches: Array<any> = e.touches; //在支持触控的设备上才有值 if (touches == undefined) return; var touchCount = touches.length; switch(touchCount) { case 1: this.stopScale(); this.beginRotation(); break; case 2: this.stopRotation(); this.beginScale(); break; default: this.stopRotation(); this.stopScale(); break; } } private onMouseUp(e:Laya.Event):void { var touches: Array<any> = e.touches; //在支持触控的设备上才有值 if (touches == undefined) return; var touchCount = touches.length; switch(touchCount) { case 1: this.stopScale(); this.beginRotation(); break; case 2: this.stopRotation(); this.beginScale(); break; default: this.stopRotation(); this.stopScale(); break; } } // 开始旋转 private beginRotation():void { this._downX = Laya.stage.mouseX; this._downY = Laya.stage.mouseY; this._rotationEnabled = true; } // 停止旋转 private stopRotation():void { this._rotationEnabled = false; } // 开始缩放 private beginScale():void { this._touchDistance = this.getTouch2Distance(); this._scaleEnabled = true; } // 停止缩放 private stopScale() { this._scaleEnabled = false; this._twoFirst = true; } // 获取两个触控点的距离 private getTouch2Distance(): number { var touchCount = this._scene.input.touchCount(); if (2 !== touchCount) return 0; var touch = this._scene.input.getTouch(0); var touch2 = this._scene.input.getTouch(1); var vec = Vector2Util.subtraction(touch2.position, touch.position); var distance = Vector2Util.getLengthSquared(vec); return distance; } public onLoop():void { if (this._rotationEnabled) { var mouseX = Laya.stage.mouseX; var mouseY = Laya.stage.mouseY; var deltaX = mouseX - this._downX; var deltaY = mouseY - this._downY; this._downX = mouseX; this._downY = mouseY; this._rotation.x = deltaY * this._factor; this._rotation.y = deltaX * this._factor; this.box.transform.rotate(this._rotation, false, false); } if (this._scaleEnabled) { if (this._twoFirst) { this._touchDistance = this.getTouch2Distance(); this._twoFirst = false; } else { var distance = this.getTouch2Distance(); var touchScale = (distance - this._touchDistance) * this._scaleFactor; this._touchDistance = distance; this._scale.x += touchScale; this._scale.y += touchScale; this._scale.z += touchScale; this.box.transform.scale = this._scale; } } } private showLog(text:string) { this.logTextArea.text += text + "\n"; } } class Vector2Util { public static getLengthSquared(vec: Laya.Vector2) { return vec.x*vec.x + vec.y*vec.y; } public static getLength(vec: Laya.Vector2): number { var s = Vector2Util.getLengthSquared(vec); return Math.sqrt(s); } public static subtraction(vec1: Laya.Vector2, vec2: Laya.Vector2) { var vec = new Laya.Vector2(); vec.x = vec1.x - vec2.x; vec.y = vec1.y - vec2.y; return vec; } public static multiply(vec: Laya.Vector2, factor: number): Laya.Vector2 { var v = new Laya.Vector2(); v.x = vec.x * factor; v.y = vec.y * factor; return v; } } class Vector3Util { public static scale(vec: Laya.Vector3, factor: number): Laya.Vector3 { var v = new Laya.Vector3(); v.x = vec.x * factor; v.y = vec.y * factor; v.z = vec.z * factor; return v; } }
运行效果
单指旋转模型,双指缩放模型
标签: LayaAir
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年11月(3)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
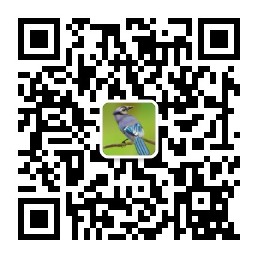