游戏资源打包
作者:追风剑情 发布于:2019-7-4 13:36 分类:Unity3d
[官方文档] AssetBundles
[官方文档] BuildPipeline
[官方文档] BuildOptions
导资源工具 (Unity 2019)
using System.Collections; using System.Collections.Generic; using System.IO; using UnityEngine; using UnityEditor; /// <summary> /// 资源工具 /// 放到Assets/Editor文件夹下 /// </summary> public class AssetsToolEditor : Editor { [MenuItem("Assets/Build/Build Model")] public static void BuildModelItem() { string export_folder = Application.streamingAssetsPath + "/models"; Object[] objects = Selection.objects; for (int i = 0; i < objects.Length; i++) BuildModel(export_folder, objects[i]); } [MenuItem("Assets/Build/Build Texture")] public static void BuildTextureItem() { string export_folder = Application.streamingAssetsPath + "/textures"; Object[] objects = Selection.objects; for (int i = 0; i < objects.Length; i++) BuildTexture(export_folder, objects[i]); } [MenuItem("Assets/Build/Build Prefab")] public static void BuildPrefabItem() { string export_folder = Application.streamingAssetsPath + "/prefabs"; Object[] objects = Selection.objects; for (int i = 0; i < objects.Length; i++) BuildPrefab(export_folder, objects[i]); } [MenuItem("Assets/Build/Build Scriptable")] public static void BuildScriptableItem() { string export_folder = Application.streamingAssetsPath + "/assets"; Object[] objects = Selection.objects; for (int i = 0; i < objects.Length; i++) BuildScriptableObject(export_folder, objects[i]); } [MenuItem("Assets/Build/Build SpriteAtlas")] public static void BuildSpriteAtlasItem() { string export_folder = Application.streamingAssetsPath + "/atlas"; Object[] objects = Selection.objects; for (int i = 0; i < objects.Length; i++) BuildSpriteAtlas(export_folder, objects[i]); } [MenuItem("Assets/Build/Build TextAsset")] public static void BuildTextAssetItem() { string export_folder = Application.streamingAssetsPath + "/text"; Object[] objects = Selection.objects; for (int i = 0; i < objects.Length; i++) BuildTextAsset(export_folder, objects[i]); } [MenuItem("Assets/Build/Build Lua")] public static void BuildLuaTextAssetItem() { string export_folder = Application.streamingAssetsPath + "/lua"; Object[] objects = Selection.objects; BuildLuaTextAsset(export_folder, objects); } [MenuItem("Assets/Build/Build Scene")] public static void BuildSceneItem() { string export_folder = Application.streamingAssetsPath + "/scene"; Object[] objects = Selection.objects; BuildSceneAsset(export_folder, objects); } // Build Model public static void BuildModel(string export_folder, Object asset) { BuildAsset(export_folder, asset, "model"); } // Build Texture public static void BuildTexture(string export_folder, Object asset) { BuildAsset(export_folder, asset, "tex"); } // Build Prefab public static void BuildPrefab(string export_folder, Object asset) { BuildAsset(export_folder, asset, "prefab"); } // Build ScriptableObject public static void BuildScriptableObject(string export_folder, Object asset) { string[] assetNames = AssetDatabase.GetDependencies(AssetDatabase.GetAssetPath(asset)); string[] assetNames1 = new string[assetNames.Length - 1]; int j = 0; for (int i = 0; i < assetNames.Length; i++) //删除依赖资源中的同名脚本,否则打包会报错。 //报错信息: Script asset "Assets/XXX/XXXX/XXXX.cs" cannot be included into AssetBundle "XXX". if (!assetNames[i].EndsWith(asset.name + ".cs")) assetNames1[j++] = assetNames[i]; BuildAsset(export_folder, asset, "asset", assetNames1); } // Build SpriteAtlas public static void BuildSpriteAtlas(string export_folder, Object asset) { BuildAsset(export_folder, asset, "atlas"); } // Build TextAsset public static void BuildTextAsset(string export_folder, Object asset) { asset.name = TrimPostfix(asset.name); BuildAsset(export_folder, asset, "txt"); } // Build Lua File public static void BuildLuaTextAsset(string export_folder, Object[] assets) { BuildAsset(export_folder, assets, "app", "lua"); } // Build Scene public static void BuildSceneAsset(string export_folder, Object[] assets) { string[] scenes = new string[assets.Length]; for (int i = 0; i < assets.Length; i++) scenes[i] = AssetDatabase.GetAssetPath(assets[i]); BuildScene(export_folder, scenes); } // 去掉文件名后缀 public static string TrimPostfix(string name) { if (string.IsNullOrEmpty(name)) return name; int index = name.IndexOf('.'); if (index == -1) return name; return name.Substring(0, index); } // 设置资源的AssetBundle信息 public static void SetAssetBundleNameAndVariant(Object asset, string bundle_name, string bundle_variant) { AssetImporter importer = AssetImporter.GetAtPath(AssetDatabase.GetAssetPath(asset)); if (importer == null) return; importer.SetAssetBundleNameAndVariant(bundle_name, bundle_variant); } // 将单个资源打成一个包 public static void BuildAsset(string export_folder, Object asset, string variant, string[] assetNames = null) { string bundleName = TrimPostfix(asset.name); Object[] assets = new Object[] { asset }; BuildAsset(export_folder, assets, bundleName, variant); } // 将一组资源打成一个包 public static void BuildAsset(string export_folder, Object[] assets, string bundleName, string variant) { if (!Directory.Exists(export_folder)) { Directory.CreateDirectory(export_folder); AssetDatabase.Refresh(ImportAssetOptions.ForceUpdate); } List<string> assetNames = new List<string>(); List<string> addressableNames = new List<string>(); for (int i=0; i<assets.Length; i++) { Object asset = assets[i]; string assetName = AssetDatabase.GetAssetPath(assets[i]); string addressableName = Path.GetFileNameWithoutExtension(assetName); assetNames.Add(assetName); addressableNames.Add(addressableName); SetAssetBundleNameAndVariant(asset, TrimPostfix(asset.name), variant); } AssetBundleBuild abb = new AssetBundleBuild(); abb.assetBundleName = bundleName; abb.assetBundleVariant = variant; //要打包到当前AB包中的资源path //加载: bundle.LoadAsset(assetNames[0]); abb.assetNames = assetNames.ToArray(); //为AB中每个资源设置昵称,方便加载 //加载:bundle.LoadAsset(addressableNames[0]); abb.addressableNames = addressableNames.ToArray(); BuildAssetBundleOptions options = BuildAssetBundleOptions.ChunkBasedCompression | BuildAssetBundleOptions.DeterministicAssetBundle; BuildPipeline.BuildAssetBundles(export_folder, new AssetBundleBuild[] { abb }, options, EditorUserBuildSettings.activeBuildTarget); AssetDatabase.Refresh(ImportAssetOptions.ForceUpdate); Debug.LogFormat("{0}/{1}.{2}", export_folder, abb.assetBundleName, abb.assetBundleVariant); DeleteManifest(export_folder, abb.assetBundleName, abb.assetBundleVariant); } // 删除生成的manifest文件 public static void DeleteManifest(string export_folder, string assetBundleName, string assetBundleVariant) { export_folder = export_folder.Substring(export_folder.LastIndexOf("/Assets/") + 1); AssetDatabase.DeleteAsset(string.Format("{0}/{1}.{2}.manifest", export_folder, assetBundleName.ToLower(), assetBundleVariant)); string folder_name = export_folder.Substring(export_folder.LastIndexOf("/") + 1); AssetDatabase.DeleteAsset(string.Format("{0}/{1}.manifest", export_folder, folder_name)); AssetDatabase.DeleteAsset(string.Format("{0}/{1}", export_folder, folder_name)); AssetDatabase.Refresh(ImportAssetOptions.ForceUpdate); } // 打包多个场景 public static void BuildScene(string export_folder, string[] scenes) { if (!Directory.Exists(export_folder)) { Directory.CreateDirectory(export_folder); AssetDatabase.Refresh(ImportAssetOptions.ForceUpdate); } foreach (string scene in scenes) BuildScene(export_folder, scene); AssetDatabase.Refresh(ImportAssetOptions.ForceUpdate); } // 打包单个场景 private static void BuildScene(string export_folder, string scene) { string assetName = Path.GetFileNameWithoutExtension(scene); string locationPathName = string.Format("{0}/{1}.scene", export_folder, assetName); BuildPlayerOptions option = new BuildPlayerOptions(); //例如 ["Assets/Scenes/Sample.unity",...] option.scenes = new string[] { scene }; option.locationPathName = locationPathName; option.target = EditorUserBuildSettings.activeBuildTarget; option.options = BuildOptions.BuildAdditionalStreamedScenes; BuildPipeline.BuildPlayer(option); Debug.Log(locationPathName); } }
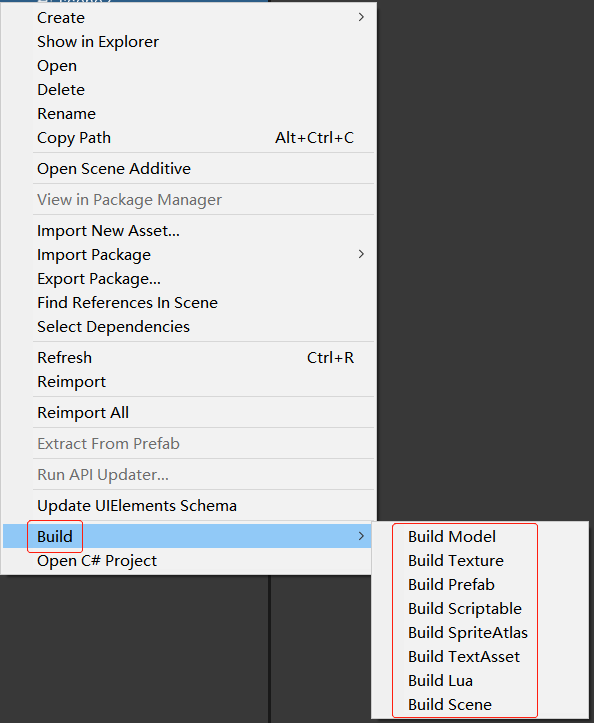
标签: Unity3d
日历
最新文章
随机文章
热门文章
分类
存档
- 2025年2月(3)
- 2025年1月(1)
- 2024年12月(5)
- 2024年11月(5)
- 2024年10月(5)
- 2024年9月(3)
- 2024年8月(3)
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
- git download
- Open CASCADE
- CascadeStudio
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
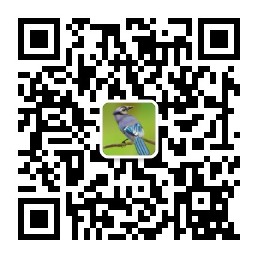